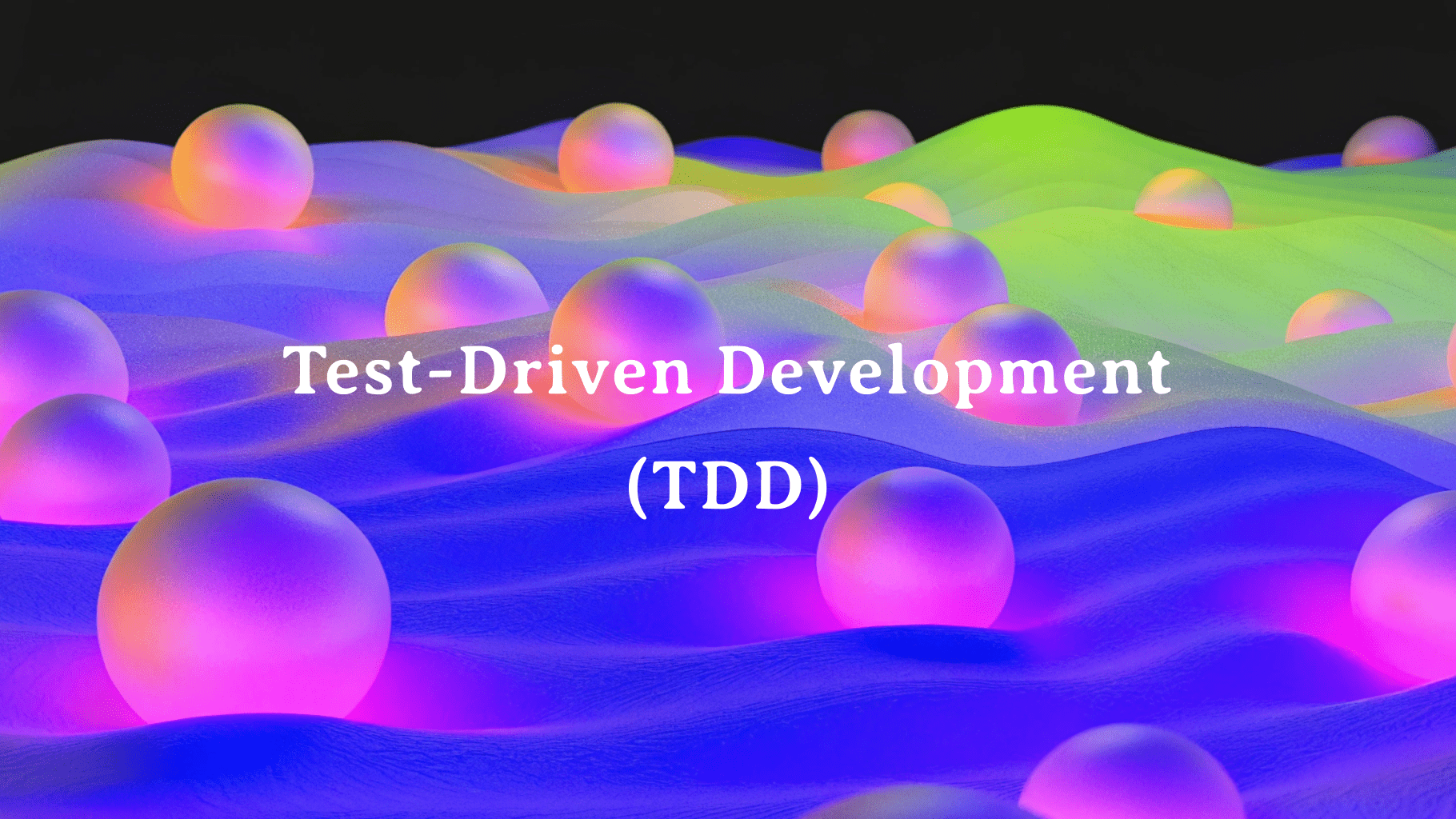
Introduction
In 2025, shipping a mobile app isn’t just about writing code that works — it’s about writing code that keeps working across an ever‑splintering device matrix, lightning‑fast OS release cadence, and user expectations of “five‑nines” reliability. Continuous Delivery pipelines get your bits into production multiple times a day; analytics and feature flags let features blossom or fail in real time; and QA automation powered by AI agents now sweeps through thousands of scenarios while you sip coffee. Yet one discipline invented a quarter‑century ago still guides teams who refuse to trade speed for quality: Test‑Driven Development (TDD) — often referred to by newcomers as “TDD testing.”
TDD is bigger than a unit testing trick. It is a feedback loop that shapes architecture, guards against regressions, and, when practiced well, shortens feedback cycles more aggressively than any late‑stage “full regression” suite ever will. By writing a failing test first — whether unit, integration testing, or contract‑level — developers codify intent before implementation. This guide distils the hard‑won lessons of teams who still meet daily release trains without drowning in flaky tests — with a special focus on mobile‑first product companies, where Quash has helped automate millions of tests every month.
Why TDD Still Matters in 2025
The quality‑speed trade‑off in mobile releases
Apple pushed eight point releases to iOS 18 in the last twelve months; Google Play’s “auto‑update” window has shrunk to hours. Without a safety net, each release becomes a game of roulette. TDD’s incremental approach catches regressions at commit time, keeping master/main reliably releasable.
The AI/agent automation wave (and where human‑written tests fit)
LLM‑powered tools now draft boilerplate tests, fuzz input ranges, and simulate flaky networks. But the intent of a feature — the thing that differentiates your product — is codified by human developers. TDD ensures that intent is written down before implementation, so AI helpers extend, rather than contradict, the engineer’s mental model.
TDD 101: Concepts & Cycle
Red ➜ Green ➜ Refactor explained
Red – Write a small failing test that expresses the next bit of behaviour.
Green – Make the simplest code change to pass. Focus on correctness, not aesthetics.
Refactor – Clean both test and production code, keeping all tests green.
Kent Beck describes this as “a minute‑by‑minute micro‑commitment to good design.” Red ensures you understand the requirement; Green proves you delivered it; Refactor buys tomorrow’s velocity.
Unit Testing vs. Integration Testing in TDD
Classic TDD favours unit scope: fast, isolated, deterministic. In mobile apps, certain seams —‑ UI bindings, database cursors, network calls — become seams for dependency inversion. Integration or contract tests then cover interactions across these seams without mocking everything.
Core Benefits (with a Mobile‑App Lens)
Fewer regressions across the OS/device matrix
Tests that exercise view‑model logic and persistence adapters catch breakages before they interact with the GPU or kernel quirks of an obscure handset.
Faster CI/CD feedback loops
A green build inside five minutes reinforces flow. Teams practicing TDD typically report 30‑50 % lower mean time‑to‑detect (MTTD) for critical failures compared with teams relying on nightly UI suites.
Improved architecture & refactor safety
When every behaviour is pinned by a test, you can swap RxJava for Kotlin Coroutines or migrate from Realm to SQLDelight with surgical confidence.
“TDD isn’t about testing. It’s about design with a safety harness.” — Martin Fowler, 2024 podcast interview
Common Myths & Anti‑Patterns
“TDD is slow”
Early iterations feel slower, but cumulative velocity rises as debugging time plummets. A 2024 Thoughtworks survey found TDD teams released 32 % more frequently than non‑TDD peers.
Over‑mocking & brittle tests
When every collaborator is mocked, refactors shred test suites. Prefer narrow seams and contract tests that exercise real integrations.
Golden snapshot obsession
Pixel‑perfect screenshots help catch layout drift, but overusing them glues tests to non‑functional chrome. Target behaviour, not implementation.
Frameworks & Tooling Stack (2025 snapshot)
Platform | Core TDD Libraries | Notes |
iOS | XCTest, Quick/Nimble, Point‑Free’s SnapshotTesting | Swift macros cut boilerplate; device cloud runners parallelise on‑device tests. |
Android | JUnit5, Espresso, Robolectric 5, Turbine for Coroutines | ART VM in Robolectric 5 matches Android 15 API changes. |
Cross‑platform (React Native / Flutter) | Jest, Detox, Playwright‑Mobile, Mockito‑dart | Flipper plugins expose JS logs to Detox for richer assertions. |
Backend / API | PyTest, Testcontainers, WireMock 3 | Containerised stubs allow local contract tests mirroring prod schemas. |
CI Orchestrators | GitHub Actions, CircleCI, Bitrise, Buildkite | Use matrix builds for OS version coverage. |
Quash AI Agents | Autogenerate negative paths, concurrency scenarios; syncs failing tests back into XCTest/JUnit suites. |
Step‑by‑Step Workflow Example
Bootstrap project
Enable code coverage thresholds (e.g., 80 %)*
Configure Quash agent for mutation test suggestions.*
Write first failing test
func test_balanceStartsAtZero() {let wallet = Wallet()XCTAssertEqual(wallet.balance, 0)}
3. Implement minimum code
struct Wallet { var balance = 0 }
4. Refactor for future features
Replace Int
with Decimal
, move state behind a protocol for DI. Tests remain green.
5. Continuous Integration
Push to main
; GitHub Action triggers unit matrix, static analysis, and Quash mutation diff. Build passes in <4 min.
TDD vs. BDD vs. ATDD (Acceptance Test-Driven Development) – Choosing the Right Approach
Axis | TDD | BDD | ATDD |
Primary author | Developer | Dev + QA + PM | Whole team |
Spec language | Code‑level assertions | Gherkin / natural language | Acceptance criteria |
Feedback speed | Milliseconds | Seconds‑minutes | Minutes‑hours |
Best when | Driving design, fast loops | Clarifying behaviour with stakeholders | Ensuring feature completeness |
Many teams layer them: core logic under TDD, high‑level flows under BDD, release acceptance under ATDD.
Expert Takeaways & Future Trends
“Clean code that works is the goal. TDD is merely the discipline that gets us there.” — Kent Beck, TDD by Example (20th anniversary edition, 2025)
AI‑assisted test authoring will draft 70 % of happy‑path tests; humans focus on edge cases and intent.
Continuous Mutation Testing surfaces weak assertions early.
DORA metrics in IDE let you see lead time, change failure rate as you type.
Key Metrics & KPIs to Track
Mean Time‑to‑Detect (MTTD) – Goal: <30 min for critical regressions
Mean Time‑to‑Resolve (MTTR) – Goal: <4 h
Test Execution Time – Keep unit suite under 5 min locally
Flake Rate – <2 % on CI
Code‑to‑Test Ratio – ~1:1 for business logic modules
Final Checklist & Action Plan
Stage | Action Item | Owner | Success Signal |
1. Kick-off | Run a 2-hour workshop on Red-Green-Refactor and unit vs integration boundaries. | Lead Engineer + QA Lead | 100 % of devs push one TDD kata PR within 24 h. |
2. Establish Seams | List critical modules & identify injection points (network, DB, UI bindings). | Architects | Dependency diagram approved in sprint planning. |
3. Coverage Baseline | Enable | Dev Ops | Build fails on <80 % unit coverage. |
4. Pilot Module | Apply TDD on a high-risk feature (e.g., payments flow) until 85 %+ coverage. | PM | Zero regression bugs in next release cycle. |
5. CI Gate | Add a fast unit-testing stage (<5 min) that blocks merge. | Dev Ops | Median PR wait time ≤15 min. |
6. Quash AI Agents | Integrate Quash to auto-generate mutation & negative-path tests. | QA Automation Eng. | +30 % test count with <2 % flake rate. |
7. Metrics Dashboard | Surface MTTD, MTTR, Flake Rate, DORA metrics in Grafana/Datadog. | Dev Ops | Weekly trend review in retro. |
8. Refactor Policy | Mandate “green bar” before refactor commits; forbid drive-by test disables. | Tech Lead | <2 % disabled tests per sprint. |
9. Continuous Improvement | Quarterly mutation-testing blitz; prune brittle mocks & snapshot bloat. | QA | Mutation score +10 pp every quarter. |
10. ROI Demo | Present live metrics drop (bugs, on-call pages) to execs after 2 quarters. | PM | Budget extension for wider TDD adoption. |
Pro Tip: Treat each item as a sprint task with clear Definition of Done; don’t move to the next stage until the previous success signal is green.
Conclusion
TDD is not a relic; it is the power tool that lets lean teams ship faster because they test first, not in spite of it. With AI augmenting monotonous test authoring, developers are freer than ever to focus on expressive intent, and Quash stands ready to amplify that loop with autonomous regression sweeps across every device your users hold.
TL;DR
Write the test, watch it fail, fix the code, then clean up.
TDD shrinks feedback cycles and guides architecture.
AI agents + solid TDD = mobile releases your users actually trust.