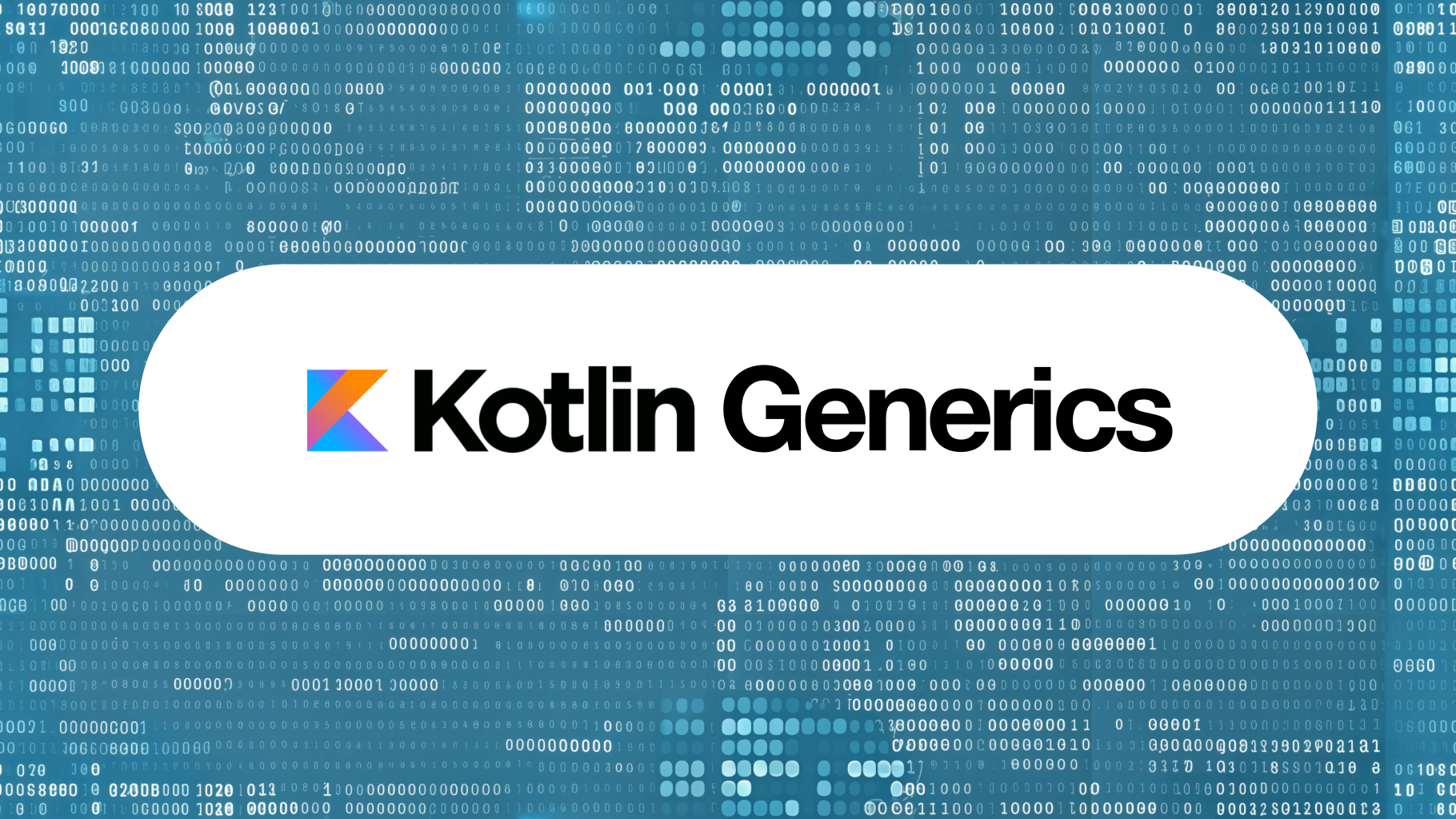
Generic programming in Kotlin provides a powerful way to write flexible, reusable code while maintaining type safety. Whether you're developing Android applications or backend services, understanding Kotlin generics is essential for writing robust and maintainable code. This comprehensive guide explores everything from basic generic types to advanced concepts like type parameters, wildcards, and reified type parameters.
Understanding Kotlin Generics: The Foundation
Kotlin generic types serve as templates that allow you to write code that works with different data types while ensuring type safety at compile time. Generic programming enables you to create classes, interfaces, and functions that can operate on different types while maintaining strong type checking.
The Power of Generic Type Parameters
Type parameters in Kotlin generics act as placeholders for actual types that will be specified later. These generic type parameters are typically represented by single letters like T
, E
, or K
, though descriptive names can be used for clarity:
// Generic class with type parameter Tclass Container<T>(private var value: T) {fun getValue(): T = valuefun setValue(newValue: T) {value = newValue}}// Using the generic Container class with different typesval stringContainer: Container<String> = Container("Hello, Kotlin Generics!")val intContainer: Container<Int> = Container(42)
Generic Interfaces and Abstract Classes
Generic classes let you define types at runtime, enabling flexibility. Generic interfaces define reusable contracts that can work with any data type. These generic interfaces are fundamental building blocks for creating flexible APIs:
// Generic interface with type parameter Tinterface Repository<T> {fun save(item: T)fun retrieve(id: String): T?fun delete(item: T)}// Implementation for a specific typeclass UserRepository : Repository<User> {override fun save(item: User) {// Implementation for saving a User}override fun retrieve(id: String): User? {// Implementation for retrieving a User}override fun delete(item: User) {// Implementation for deleting a User}}
Generic Functions and Methods
Kotlin's generic functions provide flexibility by allowing methods to handle various data types without writing separate implementations for each type. They are declared by placing type parameters in angle brackets before the function name. A generic function can have one or more type parameters, making it highly versatile. These type parameters act as placeholders for actual types that will be determined when the function is called. This approach enhances code reusability and type safety while reducing code duplication.
// Generic function with type parameter Tfun <T> printItems(items: List<T>) {items.forEach { println(it) }}// Generic method with multiple type parametersfun <K, V> createMap(key: K, value: V): Map<K, V> {return mapOf(key to value)}
Type Constraints and Bounded Type Parameters
In Kotlin, type constraints add powerful restrictions to generic type parameters, ensuring type safety and specific behavior. Using the upper bound constraint (T : Type), you can limit type parameters to specific types or their subtypes. Multiple bounds can be applied using the 'where' clause, forcing type parameters to satisfy multiple interfaces or classes simultaneously. This feature is particularly useful when you need to guarantee that generic types have certain capabilities or behaviors, like being comparable or serializable. The constraints help catch potential type-related issues at compile-time rather than runtime.
// Generic function with upper bound constraintfun <T : Number> calculateAverage(numbers: List<T>): Double {return numbers.sumOf { it.toDouble() } / numbers.size}// Generic class with multiple boundsclass DataProcessor<T> where T : Comparable<T>, T : Serializable {fun process(data: T) {// Process data that is both Comparable and Serializable}}
Advanced Generics in Kotlin: Understanding Wildcards and Type Systems
In Kotlin, generics extend beyond basic type parameters, offering sophisticated mechanisms for type-safe programming. Let's explore these concepts in detail through practical examples.
Understanding Wildcards
Wildcards in Kotlin provide flexibility when working with generic types. They're particularly useful when you want to write functions that can accept collections of various types. Here's a comprehensive look at different wildcard scenarios:
Unbounded Wildcards
These are most flexible, accepting any type. Think of them as a "don't care" type parameter:
// Generic function accepting any type of collectionfun processAnyCollection(items: List<*>) {println("Collection size: ${items.size}")items.forEach { element ->when (element) {is Number -> println("Number: $element")is String -> println("Text: $element")else -> println("Other: $element")}}}// Demonstrate flexibility with different typesfun main() {val numbers = listOf(1, 2, 3)val texts = listOf("Kotlin", "Java", "Swift")val mixed = listOf(1, "Two", 3.0)processAnyCollection(numbers)processAnyCollection(texts)processAnyCollection(mixed)}
2. Upper Bounds (out) - Covariance
When you need to read values from a collection but not modify them, upper bounds are ideal:
// Generic number processor with upper boundclass NumberProcessor {fun sumElements(numbers: List<out Number>): Double {return numbers.sumOf { it.toDouble() }}fun calculateStats(numbers: List<out Number>): String {val average = numbers.map { it.toDouble() }.average()val max = numbers.map { it.toDouble() }.maxOrNull()return "Average: $average, Maximum: $max"}}val processor = NumberProcessor()val integers = listOf(1, 2, 3)val decimals = listOf(1.5, 2.5, 3.5)println(processor.sumElements(integers)) // Works with Intprintln(processor.calculateStats(decimals)) // Works with Double
3. Lower Bounds (in) - Contravariance
Useful when you need to write values into a collection:
class AnimalShelter {// Can add any animal to a collection of its supertypefun addAnimalsTo(animals: MutableList<in Dog>) {animals.add(Dog("Buddy"))animals.add(Dog("Max"))}}open class Animal(val name: String)class Dog(name: String) : Animal(name)val animalList: MutableList<Animal> = mutableListOf()val shelter = AnimalShelter()shelter.addAnimalsTo(animalList) // Works because Animal is a supertype of Dog
Type System Features
Kotlin's type system includes powerful features for type inference and erasure:
// Type inference example with complex generic structuresclass Container<T> {fun wrap(item: T) = listOf(item)fun wrapMany(vararg items: T) = items.toList()}fun main() {// Kotlin infers types automaticallyval stringContainer = Container<String>()val wrappedText = stringContainer.wrap("Hello") // List<String>val manyTexts = stringContainer.wrapMany("A", "B") // List<String>// Type erasure in action - both are List at runtimeprintln(wrappedText::class.java) // class java.util.ArrayListprintln(manyTexts::class.java) // class java.util.ArrayList}
Best Practices for Generics in Android Development
Let's explore how to effectively use generics in Android development through practical examples that demonstrate clean, maintainable code patterns.
Smart Data Handling with Generic Collections
When working with different data types in Android, generic collections provide type safety and eliminate casting overhead. Here's how to implement a type-safe data manager:
class DataManager<T> {private val items = mutableListOf<T>()fun addItem(item: T) = items.add(item)fun getItems(): List<T> = items.toList()fun clear() = items.clear()// Generic transformation functionfun <R> transformItems(transform: (T) -> R): List<R> {return items.map(transform)}}// Usage exampleclass UserDataHandler {private val userManager = DataManager<User>()private val settingsManager = DataManager<AppSetting>()fun processUserData(user: User) {userManager.addItem(user)// Type safety ensures only User objects can be added}}
Generic UI Components
Create flexible, reusable UI components using generics. Here's an enhanced list adapter that works with any data type:
class FlexibleListAdapter<T>(private val items: List<T>,private val layoutId: Int,private val bind: (View, T, Int) -> Unit) : RecyclerView.Adapter<FlexibleListAdapter.ViewHolder>() {inner class ViewHolder(view: View) : RecyclerView.ViewHolder(view) {fun bindItem(item: T, position: Int) {bind(itemView, item, position)}}override fun onBindViewHolder(holder: ViewHolder, position: Int) {holder.bindItem(items[position], position)}}// Usageval userAdapter = FlexibleListAdapter<User>(users,R.layout.item_user) { view, user, position ->view.findViewById<TextView>(R.id.nameText).text = user.name}
Type-Safe Repository Pattern
Implement a clean repository pattern using generics for consistent data operations:
interface Repository<T> {suspend fun save(item: T): Result<T>suspend fun fetch(id: String): Result<T>}class UserRepository : Repository<User> {override suspend fun save(item: User) =runCatching { /* save implementation */ }override suspend fun fetch(id: String) =runCatching { /* fetch implementation */ }}
Understanding and Avoiding Common Generics Pitfalls in Kotlin
Let's explore the key challenges developers face when working with generics and learn practical strategies to handle them effectively. Understanding these common pitfalls will help you write more robust and maintainable code.
Runtime Type Erasure Management
One of the most significant challenges with generics is type erasure - where generic type information disappears at runtime. Here's how to handle this gracefully:
class TypeSafeContainer<T>(private val type: Class<T>) {private val items = mutableListOf<T>()fun add(item: Any) {// Safely check type before addingif (type.isInstance(item)) {@Suppress("UNCHECKED_CAST")items.add(item as T)} else {throw IllegalArgumentException("Item must be of type ${type.simpleName}")}}fun getItems(): List<T> = items.toList()}// Usage example showing type safetyfun demonstrateTypeSafety() {val stringContainer = TypeSafeContainer(String::class.java)stringContainer.add("Hello") // Works finetry {stringContainer.add(42) // Throws IllegalArgumentException} catch (e: IllegalArgumentException) {println("Cannot add Integer to String container")}}
Handling Complex Generic Structures
Instead of creating deeply nested generic types, break them down into simpler, more manageable components:
// Instead of this complex structureclass ComplexDataHolder<A, B, C> {private val nestedMap: Map<A, Map<B, List<C>>> = mapOf()}// Create a clearer hierarchydata class DataGroup<T>(val id: String, val items: List<T>)class OrganizedDataHolder<T> {private val groups = mutableListOf<DataGroup<T>>()fun addGroup(id: String, items: List<T>) {groups.add(DataGroup(id, items))}fun findInGroups(predicate: (T) -> Boolean): List<T> {return groups.flatMap { it.items }.filter(predicate)}}
Best Practices for Kotlin Generics
Meaningful Type Names: Use intuitive and descriptive names for type parameters, such as
T
for Type,E
for Element,K
for Key, orV
for Value. For complex cases, opt for fully descriptive names.Avoid Overuse: Use generics only where necessary. Overcomplicating code with excessive or deeply nested generics reduces readability and maintainability.
Documentation: Clearly explain the purpose and usage of generic classes, methods, or interfaces in comments to assist future developers.
Leverage Wildcards: Use
in
for consumers andout
for producers in generics to create flexible, type-safe APIs and simplify parameter constraints.
Benefits of Using Generics in Kotlin
Compile-Time Type Safety
Generics ensure type-safety during compilation, helping catch errors early and preventing runtime crashes.
// Without genericsval items: List<Any> = listOf(1, "hello", true)// With genericsval names: List<String> = listOf("Alice", "Bob")
2. Eliminates Explicit Casting
Generics reduce the need for manual type-casting, resulting in cleaner and safer code.
// Without genericsval numbers: List<Any> = listOf(1, 2, 3)val firstNumber = numbers[0] as Int// With genericsval numbers: List<Int> = listOf(1, 2, 3)val firstNumber = numbers[0] // No cast needed
3. Code Reusability
Generics allow you to define classes and functions that can be reused for various types.
class Box<T>(val content: T)val intBox = Box(42)val stringBox = Box("Generics are great!")
4. Improved API Flexibility
Generics help create versatile and type-safe APIs.
fun <T> printList(list: List<T>) {list.forEach { println(it) }}printList(listOf("Kotlin", "Generics"))printList(listOf(1, 2, 3))
Conclusion
Mastering Kotlin generics, from basic generic types to advanced concepts like type parameters, wildcards, and reified types, is crucial for modern Android and Kotlin development. By understanding these concepts and applying them appropriately, you can create more flexible, type-safe, and maintainable code.
Whether you're working with generic functions, implementing generic interfaces, or using type parameters in your classes, the principles and patterns discussed in this guide will help you leverage the full power of Kotlin's generic programming capabilities. Remember to consider type safety, variance, and runtime behavior when designing your generic solutions.
Keep practicing with these concepts, and you'll find yourself naturally reaching for generic programming solutions when building robust and flexible applications in Kotlin.