Updated on
|
10 min
The Five Levels of AI Automation: Transforming Software Development and Beyond
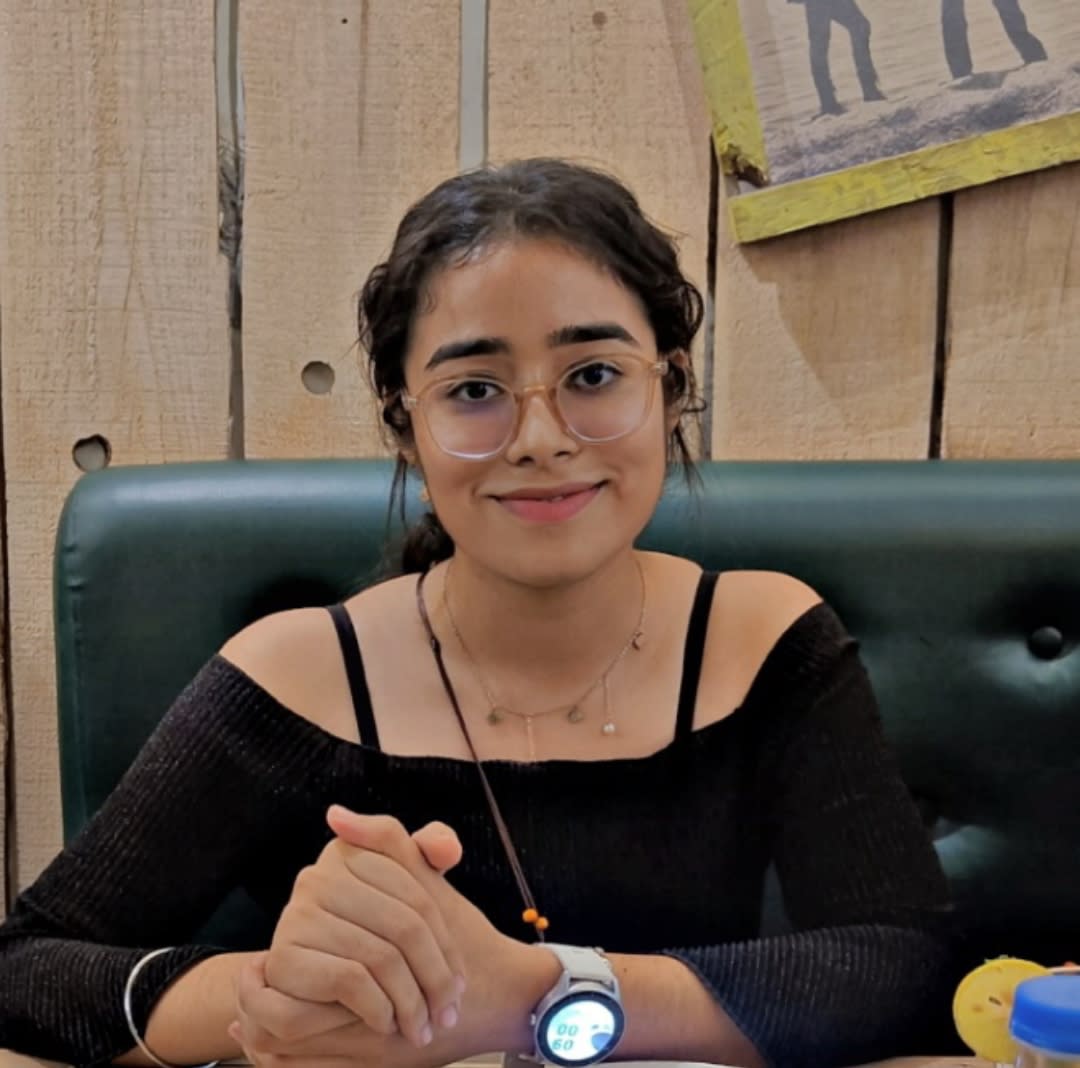
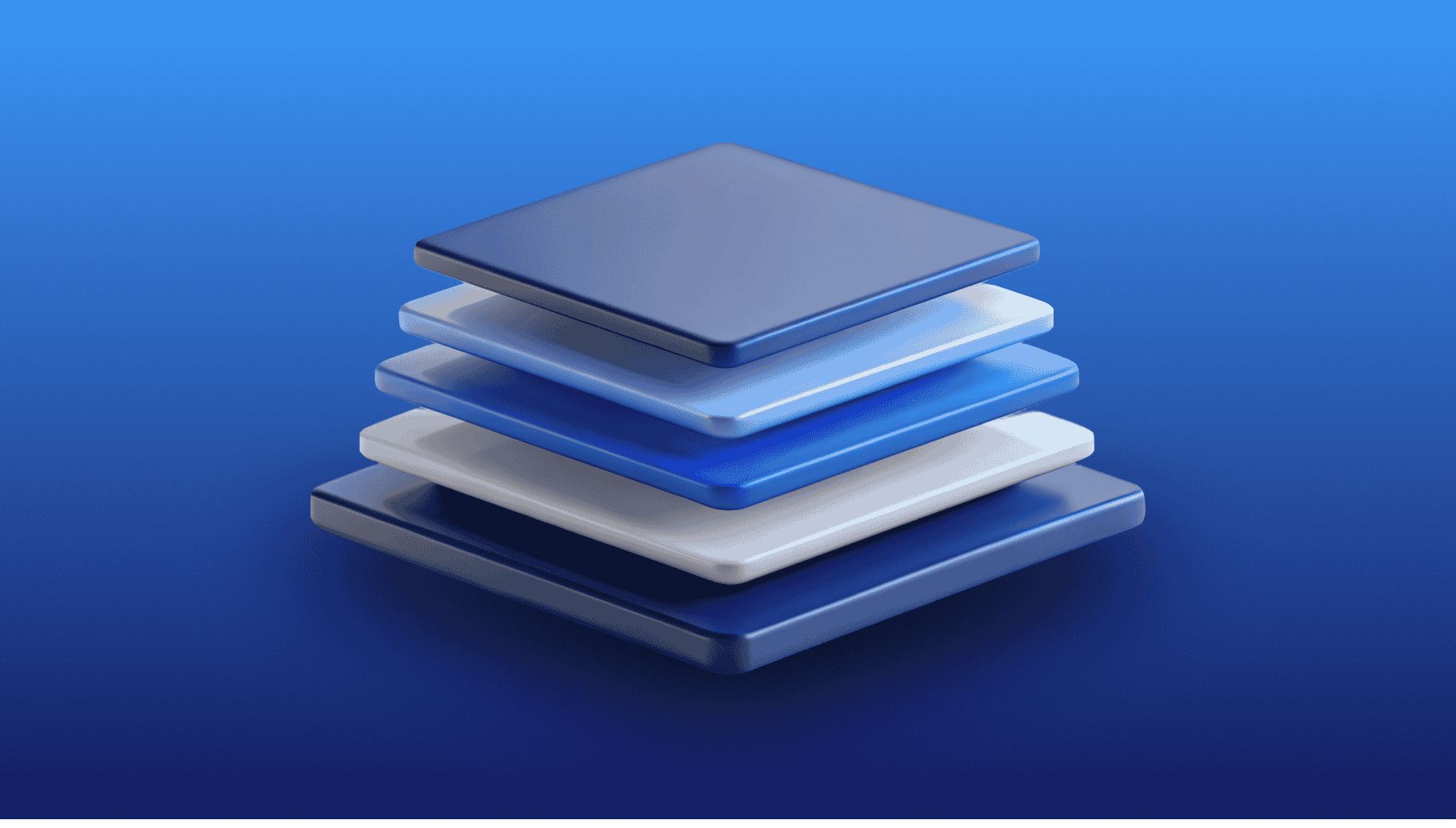
Hey fellow developers and tech enthusiasts!
Over the past decade, I've been deep in the trenches of software development, witnessing firsthand the incredible strides artificial intelligence has made in our field. Today, I want to dive into the five levels of AI automation that are not just changing how we code, but reshaping industries and our daily lives.
From Self-Driving Cars to Intelligent Code: The Journey Begins
You've probably heard about the different levels of autonomy in self-driving cars. Interestingly, that concept has made its way into software development. As someone who's navigated both traditional coding and AI integration, I've found this framework invaluable for understanding the AI revolution in our industry.
The parallels are striking. Just as autonomous vehicles are transforming transportation, AI automation is revolutionizing how we write, test, and deploy code. It's not just about easing our workload; it's about fundamentally redefining what's possible in software development.
Overview: The Five Levels of AI Automation
Before we jump in, here's a quick rundown of what we'll explore:
Rule-Based Automation : The foundation, using predefined rules and decision trees.
Self-Learning Automation : Systems that learn from experience and adjust behavior.
Limited AI Automation : Domain-specific intelligence operating autonomously within set boundaries.
Autonomous AI Automation : Handling complex, multi-faceted problems with minimal human input.
Augmented AI Automation : Seamless collaboration between AI and humans, enhancing creativity and problem-solving.

Now, let's break down each level, look at real-world examples, and see what they mean for the future of technology.
Level 1: Rule-Based Automation – The Foundation
We've all been here. This is the ground floor of our AI skyscraper, built on predefined rules and decision trees.
💡 Key Takeaway: Don't underestimate Level 1 automation. It's the springboard for advanced AI integration and can dramatically boost efficiency.
Real-World Application: CI/CD Pipelines
Think about your CI/CD pipeline. That's Level 1 in action. It's like having a tireless assistant running tests and deployments without fail.
For instance, here's a simple rule-based automation script for a CI/CD pipeline:
def run_tests(code_changes):if 'critical_module' in code_changes:run_full_test_suite()elif 'ui_component' in code_changes:run_ui_tests()else: run_quick_tests()def deploy(test_results):if test_results.all_passed:push_to_production()elif test_results.critical_tests_passedpush_to_staging()else: notify_developers()# Main pipelinecode_changes = get_latest_changes()test_results = run_tests(code_changes)deploy(test_results)
This script showcases basic rule-based decision-making in a CI/CD context—simple yet effective, forming the basis for more complex automation.
Case Study: Jenkins and Continuous Integration
I've set up Jenkins for several large-scale projects, including one for a major e-commerce platform. The impact was immediate:
Streamlined deployments
Early bug detection
Significant time savings
In one instance, we reduced deployment time from 2 hours to 15 minutes by automating our build and test processes. We broke down our monolithic application into microservices and created specialized Jenkins pipelines for each.
🔍 Developer Insight: Mastering Level 1 automation paves the way for advanced AI implementations. It's about building a solid foundation of rules and processes that can later be enhanced with machine learning.
Level 2: Self-Learning Automation – The Adaptive Learner
Now we're adding some brains to our automation. These systems learn from experience—kind of like how we learned to stop using var in JavaScript (you did stop, right?).
💡 Key Takeaway: Design robust feedback loops when implementing Level 2 systems. The quality of learning depends on the quality and diversity of the data.
Developer's Perspective: Adaptive Linters
Imagine a linter that doesn't just flag issues based on predefined rules but learns from your team's coding style over time. I've been working with such tools recently, and the impact on code consistency and review efficiency is remarkable.
Here's a conceptual example:
class AdaptiveLinter:def __init__(self):self.rules = load_initial_rules()self.team_preferences = {}def analyze_code(self, code):issues = []for rule in self.rules:if rule.check(code):issues.append(rule.generate_issue())return issuesdef learn_from_feedback(self, issue, accepted):if accepted:self.team_preferences[issue.rule] = self.team_preferences.get(issue.rule, 0) + 1else:self.team_preferences[issue.rule] = self.team_preferences.get(issue.rule, 0) - 1def update_rules(self):for rule, preference in self.team_preferences.items():if preference > THRESHOLD:self.rules.prioritize(rule)elif preference < -THRESHOLD:self.rules.deprioritize(rule)# Usagelinter = AdaptiveLinter()while True:code = get_next_code_block()issues = linter.analyze_code(code)for issue in issues:accepted = present_to_developer(issue)linter.learn_from_feedback(issue, accepted)linter.update_rules()
This example shows how a linter could adapt its rules based on team feedback, gradually aligning with your team's coding style.
Real-World Example: GitHub Copilot
GitHub Copilot takes this concept to the next level. It's not just learning from your team's code; it's learning from millions of repositories to suggest entire functions and algorithms.
My Experience:
Predicted entire functions based on context
Saved time on repetitive tasks
Stimulated coding creativity
For instance, while working on a data processing pipeline, I needed to parse a complex CSV file. I started typing:
def parse_complex_csv(file_path):# TODO: Implement CSV parsing
Copilot then suggested the following implementation:
def parse_complex_csv(file_path):result = []with open(file_path, 'r') as file:csv_reader = csv.DictReader(file)for row in csv_reader:parsed_row = {'id': int(row['id']),'name': row['name'],'value': float(row['value']),'timestamp': datetime.strptime(row['timestamp'], '%Y-%m-%d %H:%M:%S')}result.append(parsed_row)return result
This suggestion was almost exactly what I needed. With just a few tweaks to handle some edge cases, I had a working function in seconds instead of minutes.
⚠️ Ethical Consideration: Self-learning systems are only as good as their training data. Be aware of potential biases. In one project, we noticed our AI assistant suggesting variable names reflecting gender bias. We had to diversify our training data and implement bias detection in our AI model.
💡 Key Takeaway: When implementing Level 2 systems, focus on designing robust Feedback Loops. The quality of learning is directly proportional to the quality of the data it learns from. Here’s how a Feedback loop is :
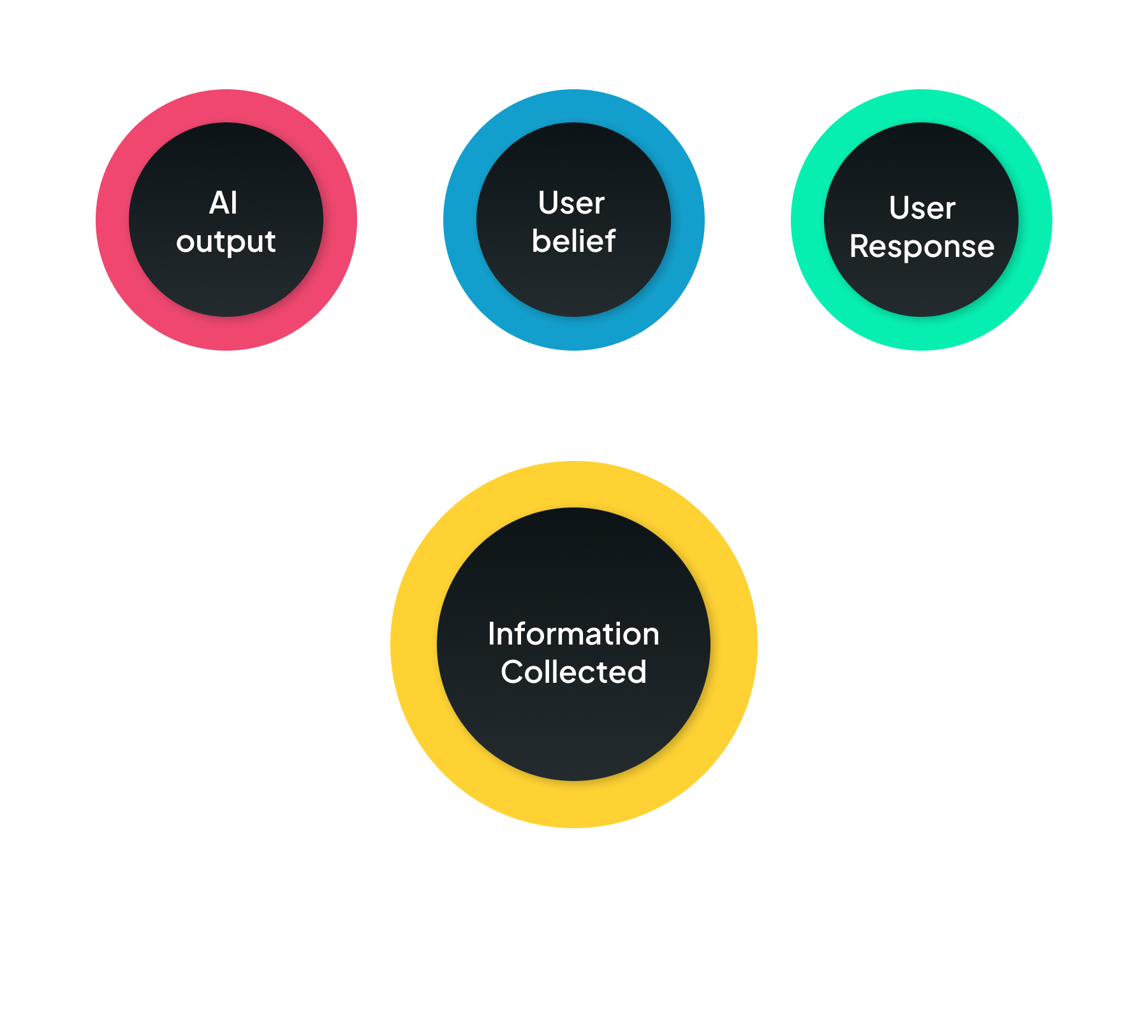
Level 3: Limited AI Automation – The Specialized Expert
At this level, AI starts to feel like that colleague who's an absolute wizard in their niche. These systems operate autonomously within well-defined boundaries.
💡 Key Takeaway: Defining operational boundaries is crucial with Level 3 automation. Be clear about what the AI can and cannot do.
Industry Example: Advanced Code Analysis Tools
Tools like SonarQube and Snyk are game-changers:
Catch critical security vulnerabilities
Provide detailed analysis and suggested fixes
Estimate potential impact of issues
Here's how a Level 3 AI might analyze code for security vulnerabilities:
class SecurityAnalyzer:def __init__(self):self.vulnerability_patterns = load_vulnerability_database()self.context_analyzer = ContextAnalyzer()def analyze_code(self, code):vulnerabilities = []for pattern in self.vulnerability_patterns:if pattern.match(code):context = self.context_analyzer.get_context(code, pattern.match_location)if self.is_true_positive(pattern, context):vulnerability = Vulnerability(pattern, context)vulnerability.suggested_fix = self.generate_fix(vulnerability)vulnerability.impact = self.estimate_impact(vulnerability)vulnerabilities.append(vulnerability)return vulnerabilitiesdef is_true_positive(self, pattern, context):# Use machine learning model to determine if this is a true positivereturn self.ml_model.predict(pattern, context)def generate_fix(self, vulnerability):# Use AI to generate a code fix based on the vulnerability and contextreturn self.fix_generator.generate(vulnerability)def estimate_impact(self, vulnerability):# Use historical data and AI to estimate the potential impactreturn self.impact_estimator.estimate(vulnerability)# Usageanalyzer = SecurityAnalyzer()vulnerabilities = analyzer.analyze_code(my_code)for v in vulnerabilities:print(f"Vulnerability found: {v.description}")print(f"Suggested fix: {v.suggested_fix}")print(f"Estimated impact: {v.impact}")
This demonstrates how a Level 3 AI goes beyond simple pattern matching to provide context-aware analysis and suggestions.
Case Study: AI in Inventory Management
While I haven't worked directly with Uber's Michelangelo, I've consulted on similar systems for:
Optimizing inventory management
Predicting demand patterns
Adapting to sudden changes in consumer behavior
In one project for a large retail chain, we implemented a system that could predict inventory needs based on historical sales data, upcoming promotions, weather forecasts, and local events.
Operational Boundaries:
Automatically adjust stock levels within a 20% range
For larger adjustments, create recommendations for human review
Not allowed to make decisions on new product introductions or discontinuations
This balance of autonomy and human oversight was key to its success.

🔍 Developer Insight: Successful Level 3 implementations involve close collaboration between developers and domain experts to set clear boundaries for AI decision-making. Be explicit about both the capabilities and limitations of the AI.
Level 4: Autonomous AI Automation – The Independent Problem Solver
Level 4 marks a significant leap. These systems handle complex, multi-faceted problems with minimal human intervention.
Cutting-Edge Application: Autonomous Testing Systems
Imagine an AI that automatically generates and executes test cases based on code changes, user behavior patterns, and historical bug data. It prioritizes tests, allocates resources efficiently, and predicts potential failure points in new features.
Real-World Glimpse: Autonomous Vehicles
While not specific to software development, autonomous vehicles like Tesla's Autopilot provide insight into Level 4 automation. They navigate complex driving scenarios, making myriad decisions every second. Similarly, in development, we're moving toward systems that can manage and create software with minimal oversight.
Consider the transformation from manual to automated deployment:
Manual Deployment:
# Manually deploying an applicationgit pull origin mainnpm installnpm run buildpm2 restart app
Automated Deployment with CI/CD Pipeline:
# GitHub Actions examplename: Deploy Applicationon:push:branches:- mainjobs:deploy:runs-on: ubuntu-lateststeps:- uses: actions/checkout@v2- name: Install dependenciesrun: npm install- name: Build applicationrun: npm run build- name: Deploy to serverrun: pm2 restart app
This mirrors how autonomous vehicles operate, and it's where development processes are heading.
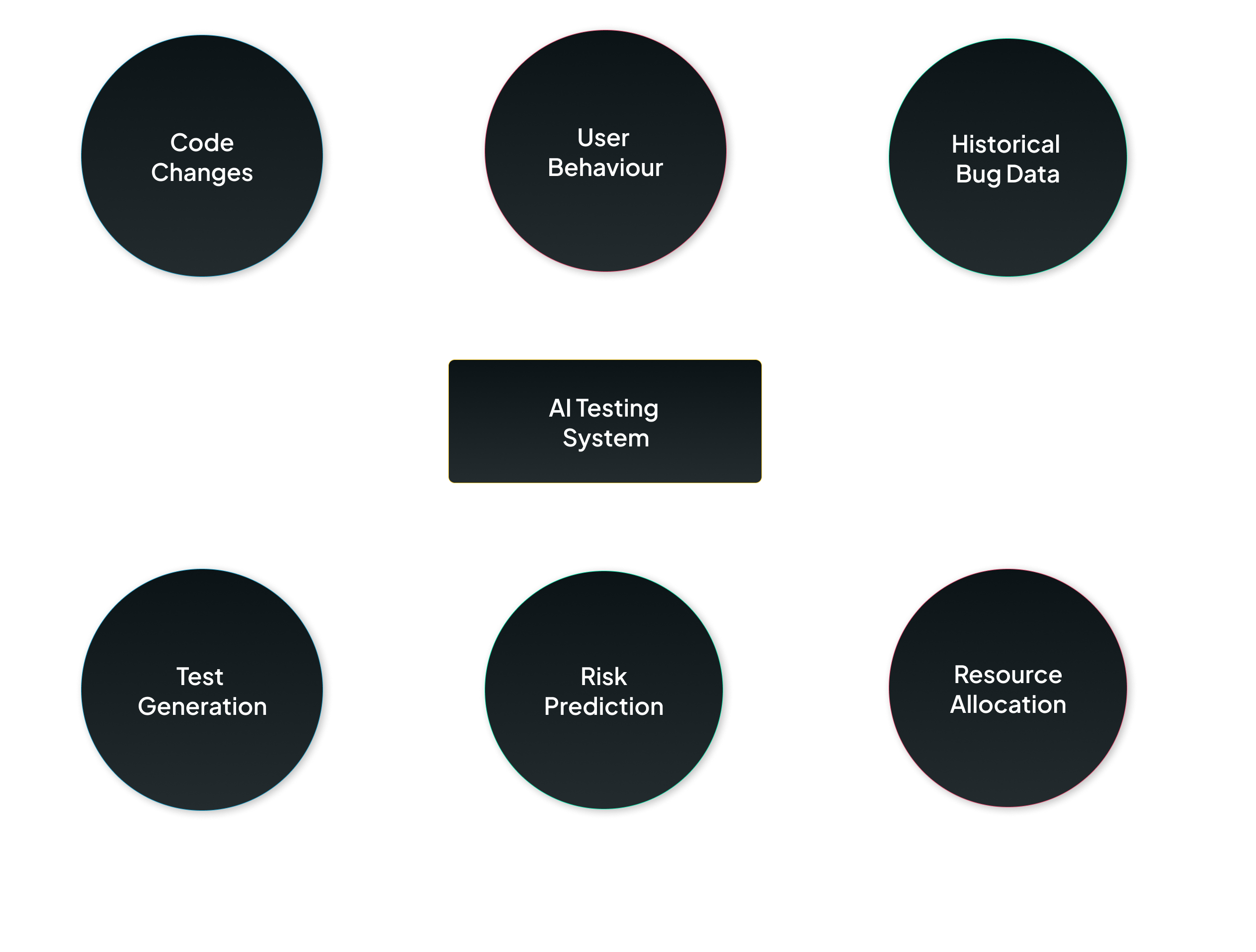
⚠️ Ethical Considerations:
Accountability : Who's responsible when an AI makes a decision leading to failure?
Transparency : How do we ensure decisions are understandable and auditable?
Economic Impact : How do we manage the transition for roles that might be automated?
These questions require ongoing dialogue among technologists, ethicists, policymakers, and society at large.
Level 5: Augmented AI Automation – The Collaborative Visionary
At the pinnacle, Level 5 systems work in seamless collaboration with human developers, enhancing creativity and problem-solving.
💡 Future Scenario: AI-Powered Software Architecture
Imagine an AI that doesn't just suggest code improvements but engages in high-level architectural discussions. It analyzes market trends, user feedback, and emerging technologies to co-create innovative solutions alongside us.
Case Study: DeepMind's AlphaFold
While not a development tool, AlphaFold represents Level 5 collaboration. By solving the protein folding problem, it's revolutionizing drug discovery and disease understanding. Imagine similar breakthroughs in software development, where AI helps solve foundational computer science problems or creates new computing paradigms.
The Human Element
As we approach Level 5, our roles evolve. Our value lies in uniquely human traits: creativity, empathy, and ethical decision-making. We're not being replaced; we're being augmented to focus on the most complex aspects of innovation.
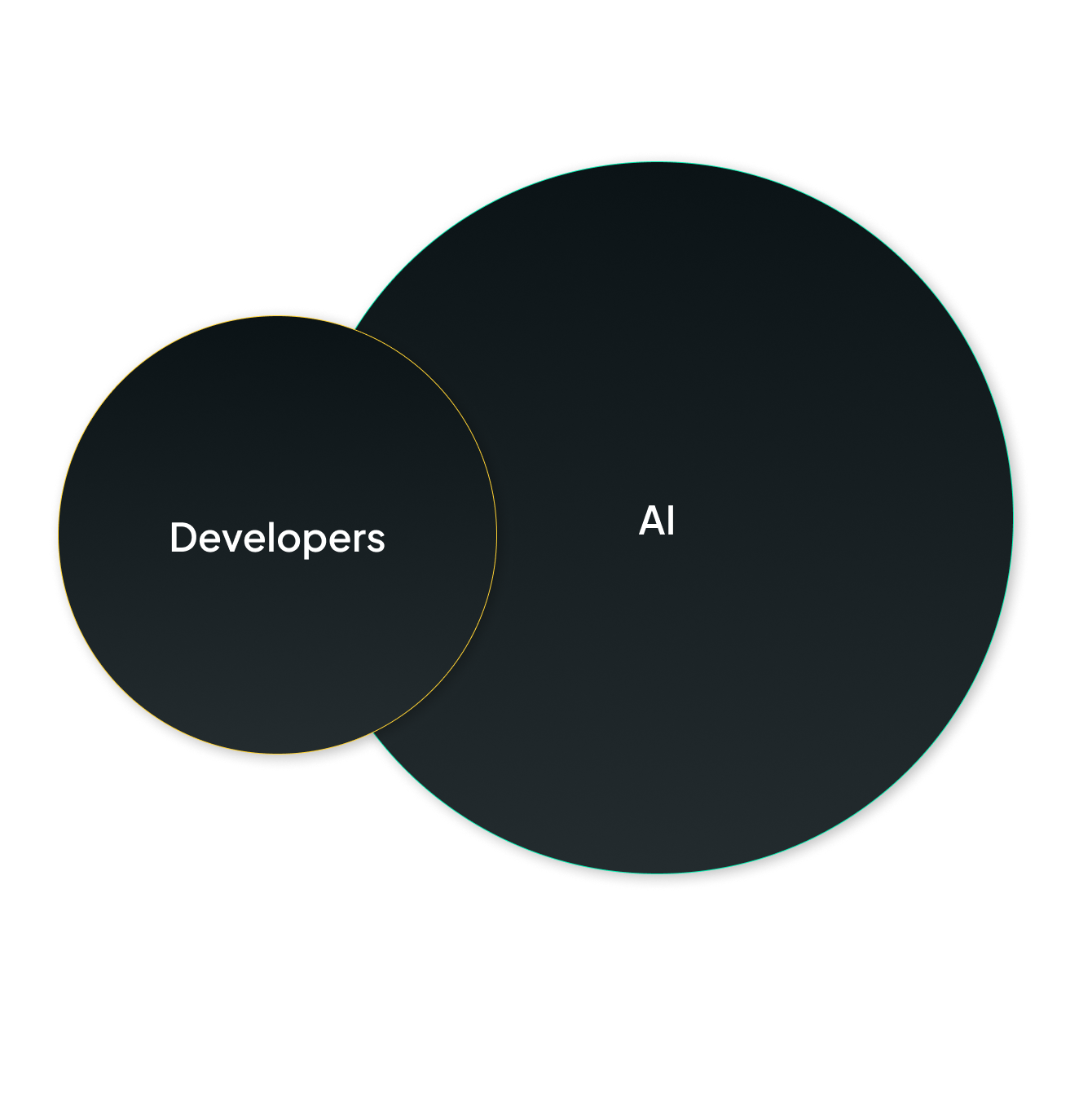
Navigating the AI-Powered Future
As we stand on the cusp of this AI revolution, it's crucial to approach these advancements with excitement and critical thinking.Key Considerations:
Continuous Learning : Stay updated on AI developments in your domain.
Ethical Mindfulness : Be vigilant about biases and ethical implications. Regular audits and diverse teams are crucial.
Human-AI Collaboration : Develop skills that complement AI capabilities—emotional intelligence, complex problem-solving, interdisciplinary thinking.
Responsible Implementation : Push for transparency, fairness, and accountability in AI systems.
Bridging the Knowledge Gap : Share AI concepts with colleagues and stakeholders. An informed team is crucial for effective integration.
Conclusion: Embracing the AI-Augmented Future
The five levels of AI automation aren't just a classification—they're a roadmap to the future of software development and innovation. As we progress, our roles will change but not diminish. AI will amplify our capabilities, allowing us to tackle more complex problems and create impactful solutions.
The question isn't whether AI will transform our industries—it's how we'll adapt and thrive. By understanding these levels and engaging with AI technologies, we can shape a future where human creativity and AI combine to push the boundaries of what's possible.
I encourage you to reflect on the current state of AI automation in your projects or industry. How do you envision it evolving? What steps can you take to prepare and shape that future? Share your thoughts and experiences in the comments below. Let's learn from each other as we navigate this exciting frontier together.
The future of technology isn't just about AI—it's about how we harness AI to enhance human potential and solve the pressing challenges of our time. Let's embrace this journey of innovation and discovery, always mindful of the profound impact our work can have on the world.
At Quash, we're excited to be part of this journey, building AI tools that empower mobile app developers and teams. We're working on creating an AI QA engineer to help you deliver better apps faster. Stay tuned for more updates!