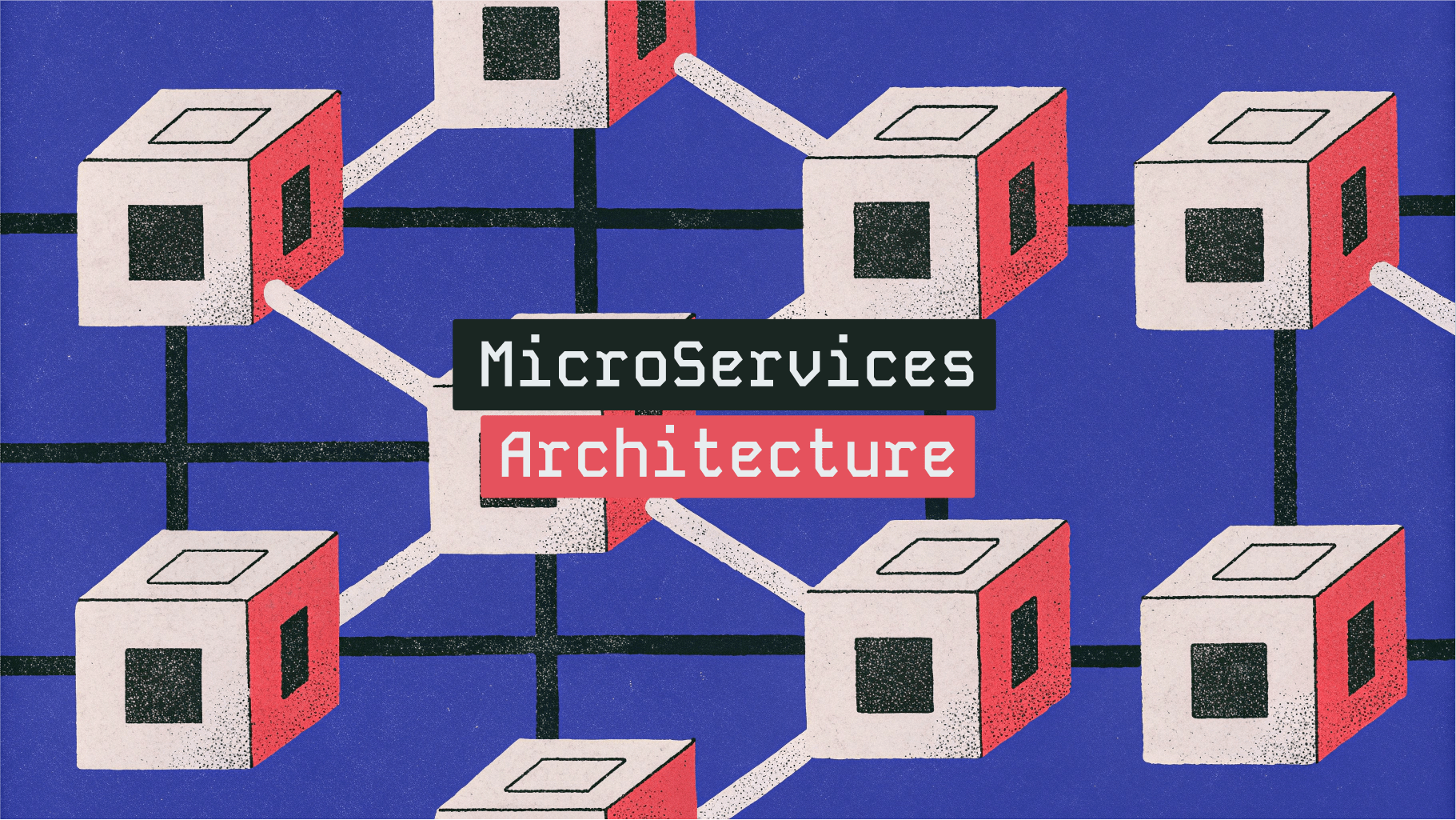
Implementing Microservices: Architecture Patterns, Best Practices, and Scaling Strategies
As modern businesses strive for faster innovation and scalability, traditional monolithic architectures often become bottlenecks. Enter microservices—a modular, flexible architecture that breaks applications into small, independent services. This paradigm shift enables teams to deploy and scale with agility, but also introduces complexities like service coordination, data consistency, and security.
In this guide, we’ll walk through key microservices architecture patterns, best practices, and strategies to help you navigate distributed systems effectively. Whether you're migrating from a monolith or starting fresh, these insights will help you build resilient and scalable microservice-based applications.
What is Microservices Architecture?
Microservices architecture is an approach to software development where an application is built as a suite of small, independent services. Each service is self-contained and communicates with others via lightweight APIs.
Key Characteristics of Microservices:
Service Independence: Each microservice runs and deploys independently.
Bounded Contexts: Services are aligned with specific business domains.
Single Responsibility: Every microservice serves a distinct purpose.
Scalability and Fault Isolation: Services scale independently and can recover from failures more easily.
Core Microservices Architecture Patterns
1. API Gateway Pattern
An API gateway acts as the single entry point for client requests. For example, an e-commerce platform may route /orders to the Order Service and /products to the Product Service.
Key Responsibilities:
Request routing and protocol translation
Authentication and rate limiting
Integration with caching and load balancing
2. Service Mesh Pattern
A service mesh handles communication between microservices, managing:
Traffic routing and observability (e.g., tracing)
Security policies (mutual TLS)
Resilience (circuit breakers, retries)
3. Database Patterns
Database per Service: Each service owns its database, promoting loose coupling.
Event Sourcing: Log state changes as events (e.g., "Order Placed") for traceability.
CQRS (Command Query Responsibility Segregation): Separate reads and writes to optimize performance.
Best Practices for Microservices Implementation
Design Principles
Loose Coupling: Reduce service interdependencies.
Smart Endpoints, Dumb Pipes: Keep logic within services, not the network.
Domain-Driven Design (DDD): Structure services around business capabilities.
Failure Resilience: Implement retries and circuit breakers.
Development Essentials
CI/CD Pipelines: Automate testing and deployment for speed.
Containerization: Use Docker and Kubernetes for consistent environments.
Monitoring and Logging: Centralized observability improves debugging.
Infrastructure as Code (IaC): Use tools like Terraform to automate infrastructure.
Security Best Practices
API Authentication: Enforce OAuth or similar protocols.
Encrypted Communication: Use TLS between services.
Network Access Control: Enforce strict policies.
Regular Audits: Identify and fix vulnerabilities.
Distributed Systems Challenges & Solutions
Data Consistency
Eventual Consistency: Accept delays where real-time syncing isn't critical.
Saga Pattern: Coordinate long-running transactions via events or orchestration.
Compensation Logic: Roll back failed operations with compensating actions.
Service Discovery & Load Balancing
Service Registry: Tools like Eureka or Consul help locate services.
Health Checks: Ensure services are reachable before routing traffic.
Load Balancers: Distribute requests across healthy instances.
Resilience & Reliability Patterns
Circuit Breakers: Prevent cascading failures by blocking failing calls.
Bulkheads: Isolate resources to contain failures.
Retries & Timeouts: Manage transient faults gracefully.
Scaling Microservices Efficiently
Horizontal Scaling
Stateless Services: Enable easy replication.
Container Orchestration: Kubernetes automates scaling and deployment.
Auto-Scaling: Dynamically adjust service instances based on load.
Vertical Scaling
Optimize CPU and memory usage.
Plan for peak loads without excessive provisioning.
Note: Scaling increases operational costs—budget accordingly.
Testing Microservices: From Unit to End-to-End
Unit Testing: Test logic in isolation using mocks.
Integration Testing: Validate service interactions and APIs.
End-to-End Testing: Simulate real-world flows, such as user transactions.
Load Testing: Ensure services perform under high traffic.
Deployment Strategies
Continuous Deployment: Use tools like Jenkins or GitHub Actions.
Blue-Green Deployments: Seamless switching between old and new versions.
Canary Releases: Gradual rollout to a subset of users.
Kubernetes Orchestration: Automate service deployment and resource management.
Performance Optimization Techniques
Caching
Use Redis or Memcached for distributed caching.
Cache API responses for frequently requested data.
Implement cache invalidation strategies.
Efficient Communication
Use REST or gRPC for synchronous needs.
Use Kafka or RabbitMQ for asynchronous, event-driven flows.
Monitoring Tools
Use Prometheus, Grafana, or Datadog for metrics.
Implement APM to detect bottlenecks in real-time.
Common Pitfalls to Avoid
Overly Granular Services: Leads to coordination overhead.
Tight Coupling: Defeats the purpose of service independence.
Weak Observability: Makes debugging and tracing difficult.
Lack of Testing: Increases the chance of regressions.
Manual Deployments: Risky and error-prone.
Future Trends in Microservices
Serverless Microservices: Run functions with minimal infrastructure.
Edge Computing: Process data closer to users for low latency.
AI & ML in Ops: Predictive scaling and anomaly detection.
Security Enhancements: Zero-trust architectures and advanced threat detection.
Protocol Advancements: Shift toward HTTP/3 and QUIC for faster communications.
Final Thoughts
Microservices offer incredible flexibility, but they come with trade-offs. Implementing the right patterns—like API Gateways, CQRS, and Sagas—alongside best practices around security, observability, and deployment is key to success.
Start small. Test the waters with a single service, containerize it, and gradually expand. With the right foundation and continuous improvement, microservices can dramatically improve how you build, scale, and maintain modern software systems.