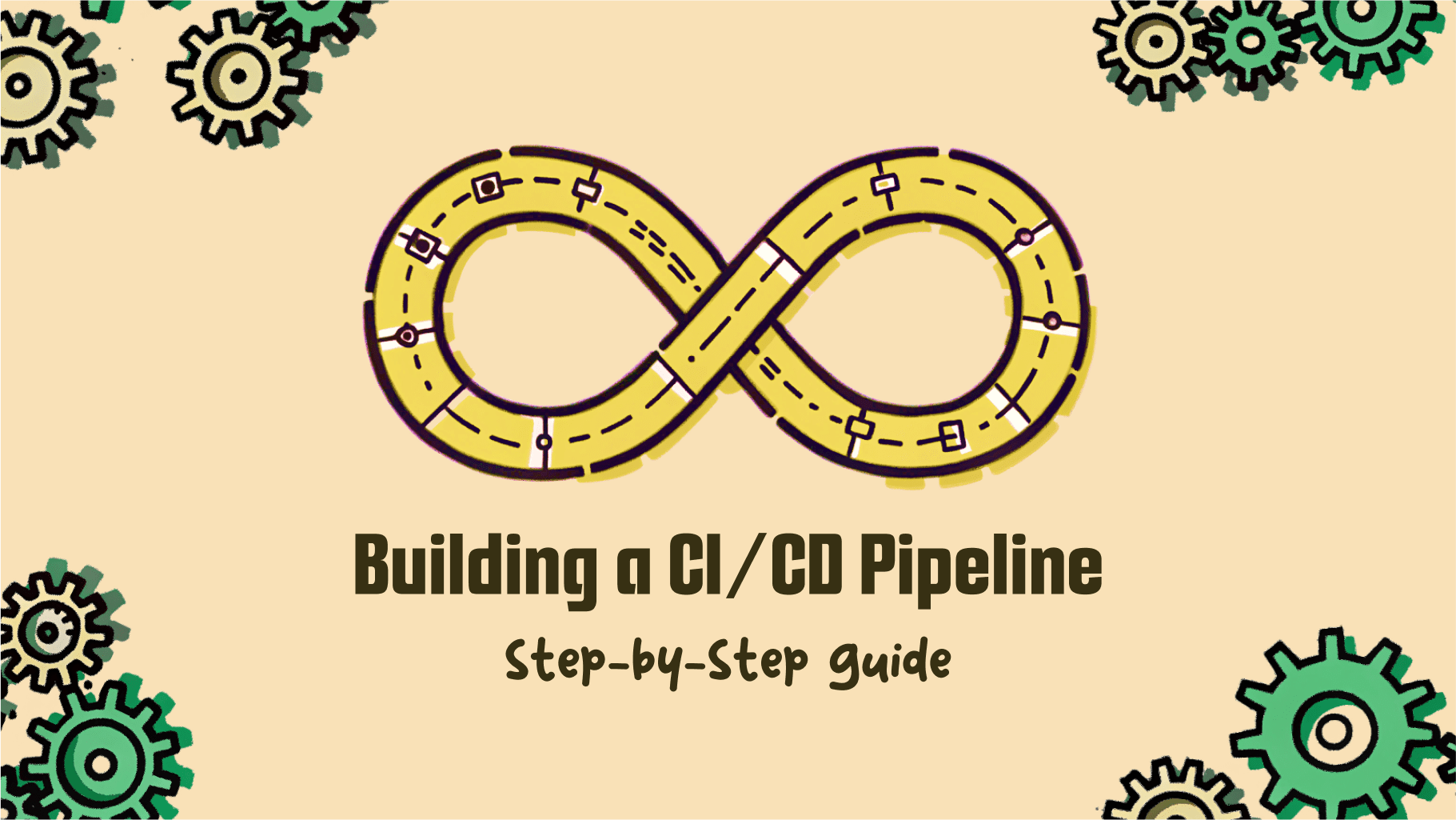
Introduction
In today’s fast-paced software development landscape, a CI/CD pipeline is essential for delivering high-quality code efficiently. By automating Continuous Integration (CI) and Continuous Delivery (CD), teams can reduce human error, improve code quality through shift left testing, and release updates more reliably. This step-by-step guide is tailored for developers and DevOps engineers with basic Git knowledge, covering fundamental concepts, automated testing, tool selection, best practices, and hands-on implementation using Jenkins and GitLab.
1. Understanding CI/CD Pipeline Fundamentals
What Is a CI/CD Pipeline?
A CI/CD pipeline orchestrates software delivery, allowing code changes to be continuously integrated, tested, and deployed. It typically includes:
Continuous Integration (CI): Automates builds and tests when developers commit updates, often incorporating shift left testing so that bugs are caught early.
Continuous Delivery (CD): Automates deployments to staging or pre-production while keeping code deployable for production.
Continuous Deployment (CD): Further extends automation to production, eliminating manual steps.
Key Benefits
Faster time to market
Improved code quality
Reduced deployment risks
Enhanced team collaboration
Consistent and reliable releases
2. Planning Your CI/CD Pipeline Architecture
Define Requirements
Identify deployment environments (e.g., dev, staging, production).
Determine testing strategies (unit, integration, end-to-end).
Address security and compliance needs (e.g., SOC 2, GDPR).
Establish monitoring and rollback mechanisms (e.g., alerts, reversion plans).
Choose Tools and Technologies
Version Control: Git (GitHub, GitLab, Bitbucket)
CI/CD Platforms: Jenkins, TeamCity, GitLab CI/CD, GitHub Actions
Testing Frameworks: JUnit (Java), Selenium (UI), Jest (JavaScript)
Containerization & Orchestration: Docker, Kubernetes
Infrastructure as Code: Terraform, Ansible
3. Setting Up Jenkins for Your CI/CD Pipeline
Jenkins Installation and Configuration
Install Jenkins on a server (e.g., Ubuntu via
apt install jenkins
).Configure security settings (e.g., LDAP or GitHub OAuth).
Install plugins like “Pipeline,” “Git,” and “Maven Integration” for build automation.
Use parallel execution by setting up multiple Jenkins build agents for faster feedback.
Creating Your First Jenkins Pipeline
Here’s a practical Jenkins pipeline for a Java Maven project:
pipeline {agent anystages {stage('Build') {steps {sh 'mvn clean package'}}stage('Test') {steps {sh 'mvn test'}post {always {junit '**/target/surefire-reports/*.xml' // Publish test results}}}stage('Deploy') {steps {sh 'scp target/my-app.jar user@prod-server:/opt/app/'}}}}
In larger environments, you can leverage parallel execution to run multiple test suites at once, reducing overall pipeline time.
4. Implementing CI/CD with GitLab
GitLab CI/CD Configuration
Create a
.gitlab-ci.yml
file in your repository root.Define pipeline stages (Build, Test, Deploy).
Configure GitLab runners (e.g., Docker-based) for automation.
Store secrets (e.g.,
$PROD_SERVER_PASSWORD
) in GitLab Settings → CI/CD.
stages:- build- test- deploybuild_job:stage: buildscript:- npm install- npm run buildartifacts:paths:- dist/test_job:stage: testscript:- npm testdeploy_job:stage: deployenvironment: productionscript:- rsync -avz --progress -e "ssh -p 22" dist/ user@$PROD_SERVER:/var/www/only:- main
5. Essential Pipeline Components
a) Source Control Integration
Branching Strategies: Use Git Flow or trunk-based development.
Code Review: Implement merge requests with approvals in GitLab.
Linting & Analysis: Enforce ESLint or SonarQube for static code analysis.
b) Automated Testing
Test Types: Unit (JUnit), integration (Postman), end-to-end (Selenium).
Parallelization: Distribute tests across multiple agents to shorten feedback loops.
Performance Testing: Use JMeter for stress or load tests.
c) Artifact Management
Store build outputs (e.g.,
.jar
, Docker images) in a registry (Nexus, GitLab container registry).Manage dependencies with Maven or npm.
Version artifacts (e.g.,
v1.0.0
).
d) Continuous Deployment Strategies
Blue-Green Deployment: Maintain two production environments for near-zero downtime.
Canary Releases: Gradually roll out changes to a subset of users.
Rolling Updates: Deploy in incremental batches to reduce disruption.
Feature Flags: Control new features with LaunchDarkly without a redeploy.
6. Security and Quality Gates
SAST: Run Checkmarx or similar in the “Test” stage.
DAST: Use OWASP ZAP post-deployment for runtime vulnerability checks.
Dependency Scanning: Integrate Dependabot or Snyk.
Container Security: Scan Docker images with Trivy.
Quality Controls
Maintain 80% code coverage with JaCoCo or Jest.
Enforce consistent standards with Prettier or Checkstyle.
Automate API doc checks with Swagger.
7. Monitoring and Optimization
Build Duration: Aim for under 10 minutes using caching and parallel execution.
Pipeline Success/Failure Rates: Track trends to identify flaky tests.
Deployment Frequency: Some teams deploy daily or multiple times per day.
Mean Time to Recovery (MTTR): Minimize recovery time with robust rollback plans.
Optimization Strategies
Distribute workloads across agents or runners for parallel processing.
Cache dependencies (e.g.,
npm ci --cache .npm
) to speed up builds.Use ephemeral cloud resources (AWS spot instances) for cost efficiency.
8. Best Practices for Managing a CI/CD Pipeline
Keep pipelines fast and efficient (under 10 minutes).
Implement fail-fast logic (e.g.,
set -e
in Bash scripts).Use environment variables for sensitive data (API keys).
Maintain pipelines as code in version control.
Schedule backups of CI/CD configs.
Document the pipeline in a README, wiki, or Confluence for easy onboarding.
9. Advanced Pipeline Features
Containerized Pipelines: Use Docker-based builds for consistency.
Cloud-Native Deployments: Target AWS ECS, Azure AKS, or GCP GKE.
IaC Automation: Provision infrastructure with Terraform in the pipeline.
Multi-Region & A/B Testing: Deploy to multiple AWS regions and test new features selectively.
Serverless CI/CD: Explore AWS Lambda or GitLab Serverless to eliminate server management.
10. Troubleshooting and Maintenance
Pipeline Failures: Check logs in Jenkins or GitLab; add retry logic (
retry: 2
in GitLab).Performance Bottlenecks: Optimize caching, parallelization, and resource allocation.
Dependency Conflicts: Pin versions in Maven, npm, or requirements files.
Environment Inconsistencies: Use Docker to keep dev, staging, and production uniform.
11. Scaling Your CI/CD Pipeline
Increase capacity by adding Jenkins agents or GitLab runners.
Load balance builds for high availability.
Integrate advanced observability with Datadog or Jaeger.
12. Future-Proofing Your Pipeline
AI/ML Test Optimization: Tools like Launchable can predict and prioritize tests.
GitOps: Use ArgoCD for declarative infrastructure management.
Progressive Delivery: Integrate solutions like Split.io for feature rollout control.
Conclusion
A well-designed CI/CD pipeline helps teams ship software faster and with greater confidence. By embracing Continuous Integration, Continuous Delivery, automated testing, and advanced deployment strategies like blue-green deployment, you’ll minimize risks and maintain high-quality releases. Start with a small, functional pipeline—perhaps using Jenkins or GitLab—and iterate with real-world feedback. Over time, add features like parallel execution, advanced security scans, and container orchestration. With a flexible, future-proofed approach, your CI/CD pipeline becomes a genuine competitive advantage, letting you deliver innovation swiftly without compromising on quality.