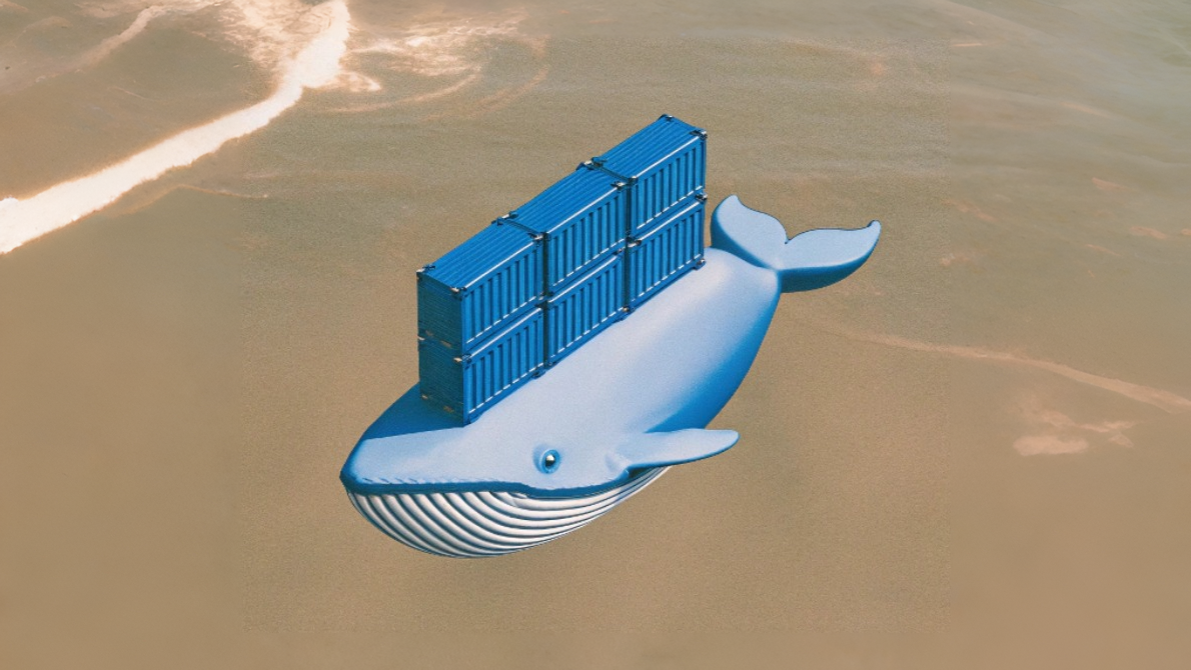
Introduction to This Docker Tutorial
In the modern software development landscape, ensuring seamless application deployment across diverse environments is more critical than ever. Containerization has emerged as the solution to the age-old problem of "it works on my machine." Among the many tools available, Docker stands out as the industry leader, transforming how developers build, package, and deploy applications. Whether you're managing standalone containers or orchestrating them in microservices architectures, Docker provides the flexibility needed for scalable applications. This guide will explore the fundamentals of Docker, its architecture, key commands, troubleshooting common issues, and best practices to help you streamline your containerized workflows. Additionally, we’ll touch on how Docker integrates with container orchestration tools like Kubernetes to optimize deployments at scale.
What is Docker?
Docker is the world's leading containerization platform, used to package applications and their dependencies into standardized units called containers. These containers ensure that your application works seamlessly across any environment—development, testing, or production.
Core Architecture
Docker Client: Interface for Docker commands
Docker Daemon: Background service managing containers
Docker Registry: Storage for Docker images
Docker Hub: The default public registry for sharing images; private registries are also supported (e.g., Docker Trusted Registry, AWS ECR, etc.)
Why Use Docker?
Development Benefits
Consistent development environments
Quick project onboarding
Simplified dependency management
Easy application sharing
Operational Benefits
Reduced infrastructure costs
Better resource utilization
Faster deployment cycles
Enhanced scalability
Business Benefits
Faster time to market
Improved application portability
Better team collaboration
Simplifies microservices development by enabling isolated, lightweight, and scalable containers
Seamless integration with Kubernetes for efficient container orchestration and scaling
Getting Started with This Docker Tutorial
Prerequisites
Before beginning with Docker, ensure your system meets these requirements:
For Windows
Windows Home users must enable WSL 2 for Docker Desktop.
Windows 10 64-bit: Pro, Enterprise, or Education (Build 16299 or later)
Hyper-V and Containers Windows features must be enabled (or use the WSL 2 backend if using Windows Home)
64-bit processor with Second Level Address Translation (SLAT)
4GB system RAM
For Mac
macOS must be version 10.15 or newer
At least 4GB of RAM
VirtualBox prior to version 4.3.30 must not be installed
For Linux
Ensure cgroups v2 is enabled, as it is often required for Docker with Kubernetes on many modern distributions.
64-bit version of Ubuntu 18.04 or newer, Debian 10 or newer, Fedora 32 or newer
Kernel version 3.10 or higher
4GB of RAM
Installation Process
Installing Docker Desktop
Download the appropriate version for your operating system
Run the installer
Follow the installation wizard prompts
Verify installation by opening terminal/command prompt and running:
docker --version docker compose --version
First Steps with Docker Tutorial
Verify Docker Installation
docker run hello-world
This command downloads a test image and runs it in a container. If successful, you'll see a welcome message.
Pull Your First Image
docker pull ubuntu:latest
This downloads the latest Ubuntu image from Docker Hub.
Run Your First Container
docker run -it ubuntu:latest
This starts an interactive shell in a new Ubuntu container.
Basic Docker Commands: A Quick Tutorial
Container Management
# List running containersdocker ps# List all containers (including stopped)docker ps -a# Stop a containerdocker stop [container_id]# Remove a containerdocker rm [container_id]
Image Management
# List imagesdocker images# Remove an imagedocker rmi [image_name]# Pull an imagedocker pull [image_name]:[tag]
Essential Docker Concepts
Docker Images
What is a Docker Image?
A Docker image is a lightweight, standalone, executable package that includes everything needed to run an application:
Code
Runtime environment
System tools
System libraries
Settings
Creating Docker Images
Images are created using a Dockerfile. Here's a basic example:
Use an official Python runtime as the base imageFROM python:3.9-slim# Set the working directory in the containerWORKDIR /app# Copy the current directory contents into the containerCOPY . /app# Install required packagesRUN pip install --no-cache-dir -r requirements.txt# Make port 80 availableEXPOSE 80# Define environment variableENV NAME World# Run app.py when the container launchesENTRYPOINT ["python", "app.py"]
Image Layers
Images are built in layers.
Each instruction in a Dockerfile creates a layer.
Layers are cached and reused.
Changes to a layer invalidate all subsequent layers.
Docker Containers
Container Lifecycle
Created: Container is created from an image
Running: Container is currently executing
Paused: Container execution is paused
Stopped: Container execution is stopped
Deleted: Container is removed
Container Commands
# Create and start a containerdocker run [options] [image]# Start an existing containerdocker start [container_id]# Pause a running containerdocker pause [container_id]# Stop a containerdocker stop [container_id]
Docker Networking
Default Networks
bridge: Default network for containers
host: Container shares host's network
none: Container has no network access
Network Commands
# Create a networkdocker network create [network_name]# List networksdocker network ls# Connect container to networkdocker network connect [network_name] [container_id]
Docker Storage
Volume Types
Named Volumes: Persistent storage managed by Docker
Bind Mounts: Direct mapping to host filesystem
tmpfs Mounts: Temporary storage in host memory
Volume Commands
# Create a volumedocker volume create [volume_name]# List volumesdocker volume ls# Inspect a volumedocker volume inspect [volume_name]
Docker Compose Tutorial
Docker Compose is a tool for defining and running multi-container Docker applications. In this Docker tutorial, we’ll focus on how Docker Compose simplifies multi-service setups.
Example docker compose.yml
version: '3.8'services:web:build: .ports:- "5000:5000"volumes:- .:/codeenvironment:FLASK_ENV: developmentredis:image: "redis:alpine"ports:- "6379:6379"
Basic Compose Commands
# Start servicesdocker compose up# Stop servicesdocker compose down# View service logsdocker compose logs# List containersdocker compose ps
Docker Compose is particularly useful for defining and running multi-container applications in development. However, for large-scale microservices deployments, tools like Kubernetes or a Docker Swarm might offer better orchestration, scaling, and management.
Docker Best Practices
Image Building
Use official base images
Implement multi-stage builds
Minimize layer count
Optimize cache usage
Security
Run containers as non-root
Scan images for vulnerabilities
Implement resource limits
Use secrets management
Performance
Optimize image size (use Alpine-based images when possible)
Implement health checks
Monitor resource usage
Use volume mounts efficiently
Docker in DevOps
CI/CD Integration
Automated builds (including GitHub Actions/Docker BuildKit)
Container registry integration
Deployment automation
Testing in containers
Container Orchestration
Docker Swarm basics
Kubernetes integration (sometimes referred to as a "Docker Kubernetes tutorial")
Scaling strategies
High availability setup
Troubleshooting Common Docker Issues
Container Startup Issues
Container Fails to Start
Symptoms:
Container exits immediately
Status shows "Exited (1)" or other non-zero exit code
Container appears in
docker ps -a
but not indocker ps
Diagnostic Steps:
docker logs [container_id]docker logs --tail 50 [container_id] # Last 50 linesdocker inspect [container_id]docker stats [container_id]
Common Solutions:
Verify the
CMD
/ENTRYPOINT
in DockerfileCheck for required environment variables
Ensure sufficient system resources
Validate volume mount permissions
Container Hangs During Startup
Symptoms:
Container status stays at "Created" or "Starting"
No response from container
High CPU or memory usage
Solutions:
# Force stop the containerdocker stop [container_id]# Remove the containerdocker rm [container_id]# Start with debug modedocker run --debug [image_name]
Network Issues
Container Network Connectivity
Symptoms:
Containers can't communicate
DNS resolution failures
Port mapping issues
Diagnostic Commands:
docker network lsdocker network inspect [network_name]docker port [container_id]docker exec [container_id] ping [destination]
Common Solutions:
1. Reset Docker networking:
# On Linuxsudo systemctl restart docker# On Windows# Restart Docker Desktop
2. Recreate network:
docker network rm [network_name]docker network create [network_name]
Port Binding Conflicts
Symptoms:
"Port is already in use" error
Container fails to start with port error
Solutions:
# Check ports in usenetstat -tulpn | grep LISTEN # Linuxnetstat -ano | findstr "LISTENING" # Windows# Change port mappingdocker run -p 8080:80 [image_name]
Storage and Volume Issues
Volume Mount Problems
Symptoms:
Missing data in volumes
Permission denied errors
Volume not mounting
Diagnostic Steps:
docker volume lsdocker volume inspect [volume_name]docker inspect -f '{{ .Mounts }}' [container_id]
Solutions:
1. Check permissions:
# Fix permissions on hostsudo chown -R user:group /path/to/volume# Mount with correct permissionsdocker run -v /host/path:/container/path:rw [image_name]
2. Clean up volumes:
# Remove unused volumesdocker volume prune# Force remove volumedocker volume rm -f [volume_name]
Resource Constraints
Memory Issues
Symptoms:
Container crashes with OOM (Out of Memory)
Performance degradation
System becomes unresponsive
Diagnostic Commands:
docker statsdocker inspect -f '{{.HostConfig.Memory}}' [container_id]
Solutions:
# Run with memory limitdocker run --memory=2g --memory-swap=2g [image_name]
Monitor and adjust:
docker run --memory=2g --memory-reservation=1.5g [image_name]
CPU Issues
Symptoms:
High CPU usage
Container performance issues
System slowdown
Solutions:
# Limit CPU usagedocker run --cpus=".5" [image_name]docker run --cpu-shares=512 [image_name]
Image Issues
Image Pull Failures
Symptoms:
"Image not found" error
Repository access issues
Network timeout during pull
Solutions:
# Force new pulldocker pull --force [image_name]# Check Docker Hub statusdocker login# Use specific tagdocker pull [image_name]:[specific_tag]
Image Build Failures
Symptoms:
Build process fails
Layer caching issues
Dependencies fail to install
Solutions:
# Build without cachedocker build --no-cache -t [image_name] .# View build stepsdocker build --progress=plain -t [image_name] .# Verify files included in builddocker build -t [image_name] . -f Dockerfile --progress=plain
Logging and Debugging
Logging Best Practices
# View real-time logsdocker logs -f [container_id]# View logs with timestampsdocker logs -t [container_id]# Limit log outputdocker logs --tail 100 [container_id]
Debug Mode
# Run a container with debug loggingdocker run --log-level debug [image_name]# Enable debug mode on the Docker daemondockerd --debug
Quick Reference: Common Commands for Troubleshooting
# Container Troubleshootingdocker ps -a # List all containersdocker inspect # Container detailsdocker logs # Container logsdocker stats # Resource usage# Network Troubleshootingdocker network ls # List networksdocker network inspect # Network detailsdocker port # Port mappings# Volume Troubleshootingdocker volume ls # List volumesdocker volume inspect # Volume detailsdocker system df # Storage usage# Image Troubleshootingdocker images # List imagesdocker history # Image layer historydocker build --no-cache # Clean build
Container Orchestration (Docker Swarm and Docker Kubernetes Tutorial Overview)
As applications grow in complexity, managing individual containers manually becomes inefficient. This is where container orchestration comes in—automating the deployment, scaling, and management of containers across multiple hosts.
While Docker is excellent for running and managing individual containers, it typically relies on additional tools for large-scale orchestration. Kubernetes is the industry-standard platform for automating container deployment, scaling, and management—often using containerd or other CRI-compatible runtimes under the hood.
Kubernetes Benefits
Automated Deployment & Scaling: Define the desired state of your application, and Kubernetes ensures it runs as expected, automatically scaling based on demand.
Self-Healing: If a container crashes, Kubernetes detects the failure and replaces it without manual intervention.
Efficient Resource Utilization: Kubernetes intelligently distributes workloads across nodes, optimizing performance and resource consumption.
For teams managing microservices architectures, Kubernetes simplifies operations by abstracting infrastructure complexities and enabling seamless container orchestration. While Docker provides the foundation for containerized applications, Kubernetes ensures they run efficiently in production environments.
Conclusion
Docker has fundamentally changed how we build and deploy applications. Whether you're just starting with containerization or looking to optimize your workflows, mastering Docker’s core concepts and best practices is essential. By following the practices and concepts covered in this guide, you'll be well-equipped to:
Build efficient containerized applications
Implement Docker in your development workflow
Troubleshoot common issues
Scale your applications effectively
As your containerized applications grow, Docker’s integration with orchestration tools like Kubernetes opens new possibilities for scaling and automation. Together, they provide a solid foundation for building resilient, portable applications that can seamlessly adapt to your evolving needs.