
Introduction
In Android development, being efficient is crucial. I remember starting on a big e-commerce project back in 2015 and being struck by how slow our build times were. Those long waits were more than just annoying—they really held back our team's work. In this post, I want to share some advanced Gradle techniques I've learned over the years. These strategies have helped me speed up build times significantly, making our projects run smoother and letting our team stay focused and productive.
1. Optimizing Build Times
The True Cost of Slow Builds
The frustration of waiting for a build to complete is familiar to you if you've worked on large-scale Android projects. Slow builds do more than just waste time—they disrupt your workflow, dilute your focus, and can drastically reduce your productivity. In one of my earlier projects, an e-commerce platform, we initially faced 15-minute build times. For our team of 20 developers, this delay was a significant productivity killer.
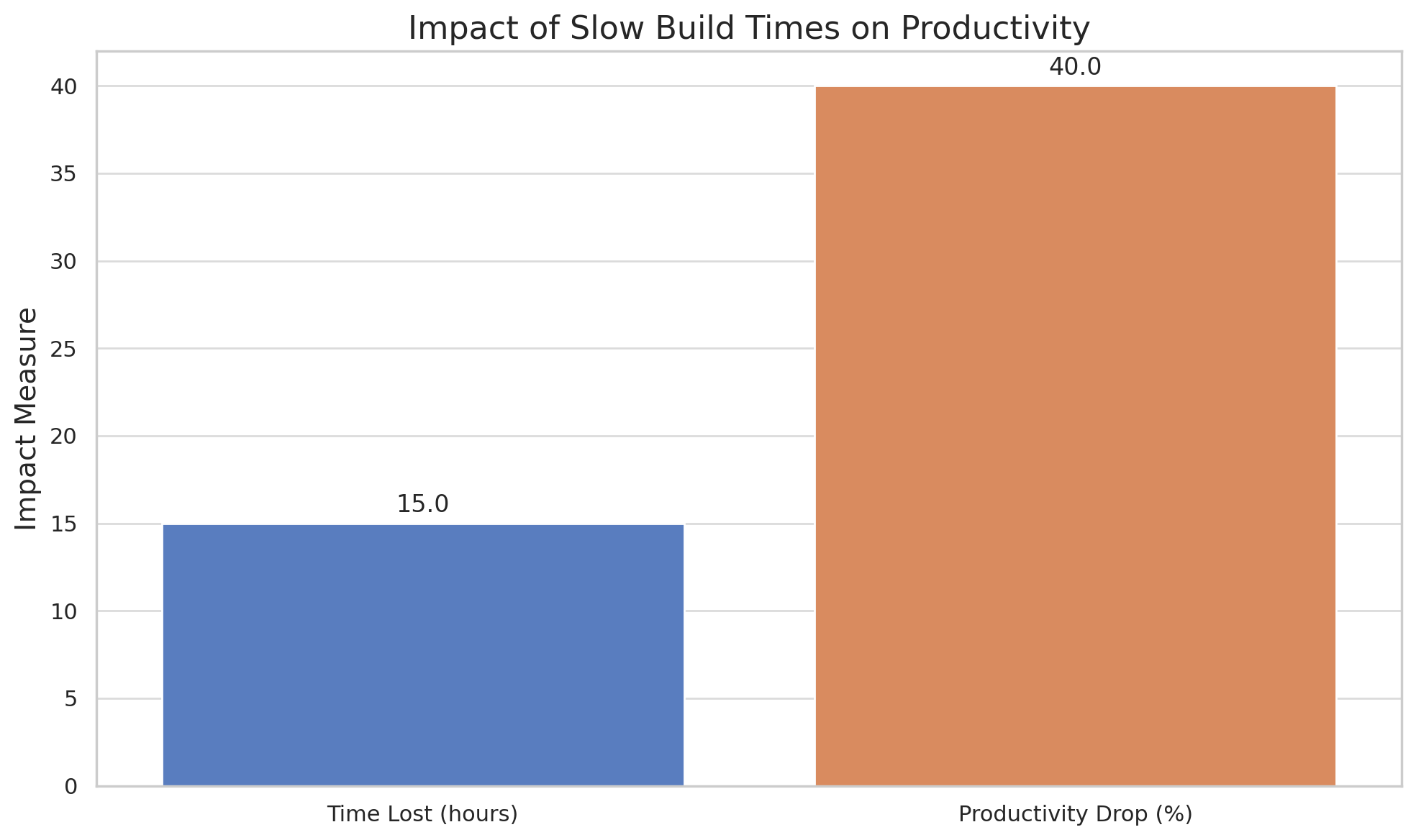
Strategies to Accelerate Gradle Builds:
Enabling Parallel Execution and Effective Caching
To combat these slow build times, significant modifications to the gradle.properties and root build.gradle files were necessary to enhance our build efficiency:
properties
# gradle.propertiesorg.gradle.parallel=trueorg.gradle.caching=trueorg.gradle.configureondemand=true
groovy
# root build.gradleallprojects {tasks.withType(JavaCompile) {options.fork = trueoptions.incremental = true}}
Actionable Step
To immediately benefit from these optimizations, start by integrating the org.gradle.parallel and org.gradle.caching properties into your gradle.properties file today. Monitor your build times before and after these changes to directly observe the impact and tweak further as needed.
These tweaks allowed us to run builds in parallel, leveraging module independence to drastically reduce waiting times. By introducing effective caching, we managed to cut our build times by about 40%. This change meant that you could iterate faster and more efficiently, significantly boosting your team's output and morale.
Maximizing Build Cache Utilization
To further enhance our build speeds, we implemented a shared build cache on our CI server:
groovy
# build.gradlebuildCache {local {directory = new File(rootDir, 'build-cache')removeUnusedEntriesAfterDays = 30}remote(HttpBuildCache) {url = 'https://example.com/cache/'credentials {username = 'username'password = 'password'}push = true}}
This configuration enabled you to leverage cached builds across the team, dramatically speeding up both clean builds and routine checks. By reusing previously compiled code, the need for redundant compilations was minimized, offering a significant time saving. For instance, on another project, a healthcare app with over 30 modules, implementing a shared build cache reduced our CI build times from over an hour to just under 30 minutes.
Incorporating these advanced Gradle techniques transformed the build process in my projects. By optimizing build times, not only did we enhance developer satisfaction and efficiency, but we also managed to reduce costs associated with delayed timelines and developer downtime. This approach has proven invaluable, and I encourage you to adapt these strategies to fit the specific needs of your projects for optimal results.
2. Implementing Custom Gradle Plugins
Customizing Your Build Processes
As the scale of our projects increased, so did the need for consistent and repeatable build tasks, particularly for code quality checks. To address this, we turned to custom Gradle plugins, which proved to be a robust solution for automating and standardizing these processes across all modules.
Developing and Integrating a Custom Plugin
The journey began with setting up a new Gradle plugin project. Our goal was to create a simple yet effective plugin to automate Kotlin's code quality checks. Here's how we did it:
kotlin
// buildSrc/src/main/kotlin/CodeQualityPlugin.ktimport org.gradle.api.Pluginimport org.gradle.api.Projectclass CodeQualityPlugin : Plugin<Project> {override fun apply(project: Project) {project.tasks.register("runCodeQuality") {doLast {project.exec {commandLine("./gradlew", "ktlint", "detekt")}}}}}
Actionable Step
Once you've developed your custom plugin, ensure it's robust by testing it in a controlled environment before rolling it out across all modules. This step is crucial for ensuring that your custom solutions integrate seamlessly into your development workflow without introducing new issues.
After refining this plugin, we published it to a Maven repository to make it easily accessible. Integrating the plugin into our projects was straightforward:
groovy
// build.gradleplugins {id 'com.example.codequality' version '1.0'}
This plugin significantly streamlined our code quality processes, ensuring consistency across all development modules without manual intervention.
3.Effective Dependency Management in Multi-Module Projects
Streamlining Dependencies
Managing dependencies efficiently in large projects can be incredibly complex, as we discovered while working on a healthcare application with over 30 modules. This complexity can lead to version conflicts, increased build times, and maintenance headaches. To address these issues, we adopted a centralized approach to dependency management.
Centralized Dependency Management
To simplify dependency management, we created a dependencies.gradle file. This file serves as a single source of truth for all dependency versions and references, ensuring consistency across the entire project.
Example dependencies.gradle
File:
groovy
// dependencies.gradleext {versions = [kotlin : '1.5.0',androidxCore : '1.3.2',// more versions]deps = [kotlin : "org.jetbrains.kotlin:kotlin-stdlib:${versions.kotlin}",androidxCore : "androidx.core:core-ktx:${versions.androidxCore}",// more dependencies]}
This centralized file is then applied across all modules, allowing us to maintain a cohesive and up-to-date list of dependencies. By doing this, we ensure that all modules use the same versions of each dependency, which reduces the risk of version conflicts and simplifies the update process.
Applying dependencies.gradle in Module build.gradle Files:
groovy
// module build.gradleapply from: "$rootProject.projectDir/dependencies.gradle"dependencies {implementation deps.kotlinimplementation deps.androidxCore// more dependencies}
By implementing centralized dependency management, we were able to streamline our development process, reduce build times, and maintain consistency across our project. This approach has proven invaluable in managing the complexities of large, multi-module projects.
Case Study: Transforming Build Dynamics
Let me share a story from when we were working on a large social media app with over 50 parts. Initially, our big, continuous integration (CI) builds took more than an hour, and even the smaller, local builds were quite slow. We managed to reduce the CI build times significantly by running builds in parallel and using custom plugins. However, the biggest change came when we set up a centralized system to handle our dependencies.
By using these strategies, we saw big improvements:
Build Time Reduction: Our CI build times went down from 65 minutes to just 22 minutes, and our local clean builds fell from 25 minutes to 8 minutes.
Productivity Impact: Faster build times meant we could work faster, catch code issues earlier, and shorten the time it took to release updates.
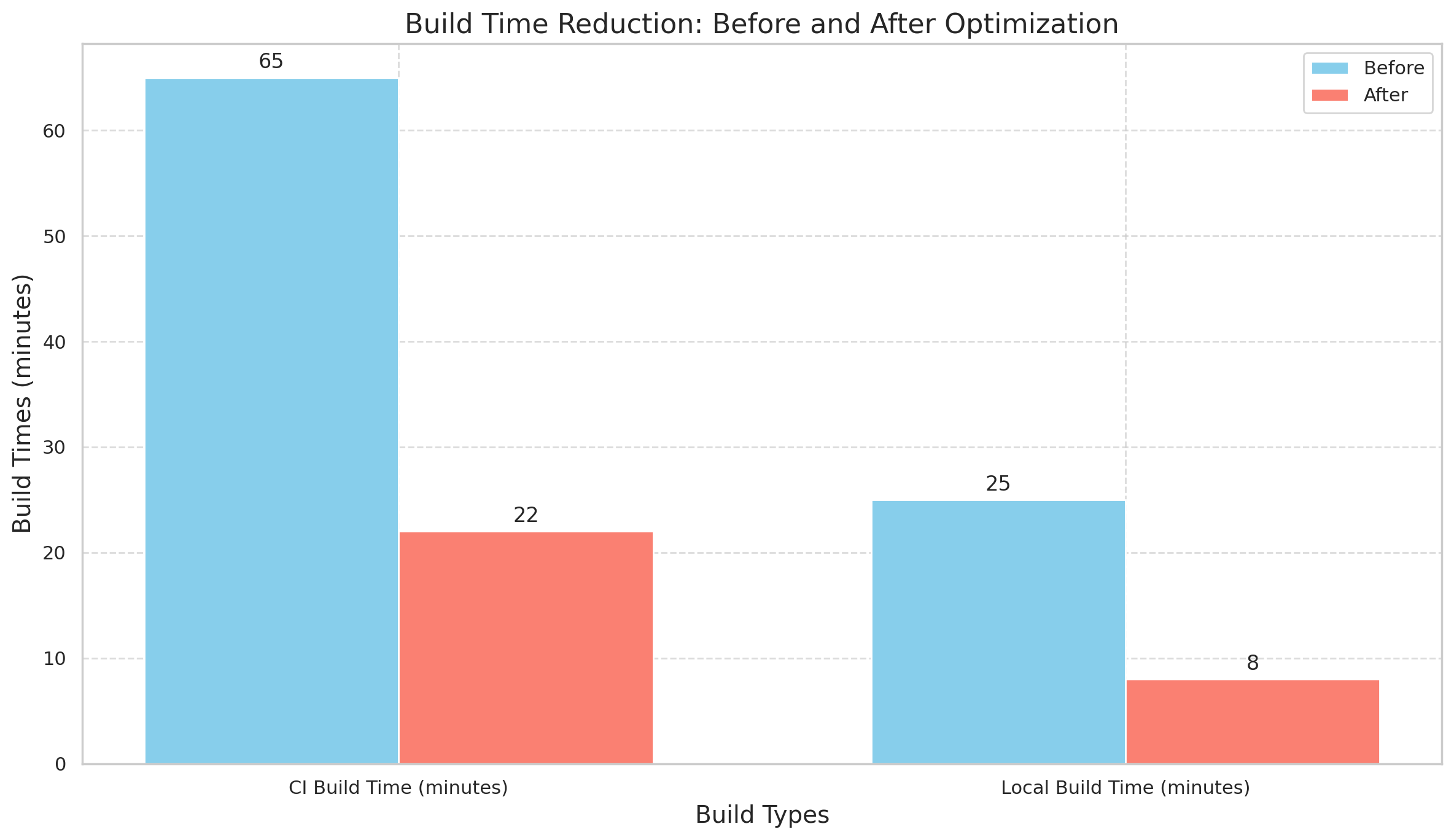
These changes not only made our development team more efficient but also boosted the overall momentum and satisfaction of the project. By continually improving these processes, you can get the best results tailored to the needs of your projects.
Conclusion
Mastering advanced Gradle techniques is more than a technical necessity—it's a strategic advantage for any developer facing the challenges of large-scale Android projects. The strategies we've discussed have not only been tested in the trenches of real-world applications but have also consistently demonstrated their value in enhancing both the speed and quality of development.
As we continue to push the boundaries of what's possible with Android development, I'm eager to learn from your experiences as well. Have you tried implementing any of these advanced techniques in your projects? What unique challenges have you encountered, and how have you adapted these strategies to meet your needs? Please share your insights, questions, or even your own success stories in the comments below. Let's cultivate a community where we can all grow and refine our practices together.
Let’s collaborate and elevate our Android projects to new heights!